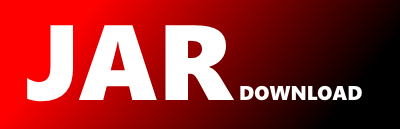
com.bumptech.glide.load.resource.transcode.DrawableBytesTranscoder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of glide Show documentation
Show all versions of glide Show documentation
A fast and efficient image loading library for Android focused on smooth scrolling.
The newest version!
package com.bumptech.glide.load.resource.transcode;
import android.graphics.Bitmap;
import android.graphics.drawable.BitmapDrawable;
import android.graphics.drawable.Drawable;
import androidx.annotation.NonNull;
import androidx.annotation.Nullable;
import com.bumptech.glide.load.Options;
import com.bumptech.glide.load.engine.Resource;
import com.bumptech.glide.load.engine.bitmap_recycle.BitmapPool;
import com.bumptech.glide.load.resource.bitmap.BitmapResource;
import com.bumptech.glide.load.resource.gif.GifDrawable;
/**
* Obtains {@code byte[]} from {@link BitmapDrawable}s by delegating to a {@link ResourceTranscoder}
* for {@link Bitmap}s to {@code byte[]}s.
*/
public final class DrawableBytesTranscoder implements ResourceTranscoder {
private final BitmapPool bitmapPool;
private final ResourceTranscoder bitmapBytesTranscoder;
private final ResourceTranscoder gifDrawableBytesTranscoder;
public DrawableBytesTranscoder(
@NonNull BitmapPool bitmapPool,
@NonNull ResourceTranscoder bitmapBytesTranscoder,
@NonNull ResourceTranscoder gifDrawableBytesTranscoder) {
this.bitmapPool = bitmapPool;
this.bitmapBytesTranscoder = bitmapBytesTranscoder;
this.gifDrawableBytesTranscoder = gifDrawableBytesTranscoder;
}
@Nullable
@Override
public Resource transcode(
@NonNull Resource toTranscode, @NonNull Options options) {
Drawable drawable = toTranscode.get();
if (drawable instanceof BitmapDrawable) {
return bitmapBytesTranscoder.transcode(
BitmapResource.obtain(((BitmapDrawable) drawable).getBitmap(), bitmapPool), options);
} else if (drawable instanceof GifDrawable) {
return gifDrawableBytesTranscoder.transcode(toGifDrawableResource(toTranscode), options);
}
return null;
}
@SuppressWarnings("unchecked")
@NonNull
private static Resource toGifDrawableResource(@NonNull Resource resource) {
return (Resource) (Resource>) resource;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy