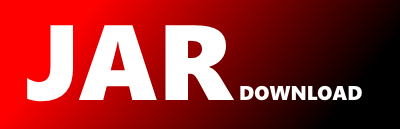
com.github.chaosfirebolt.converter.api.cache.DefaultCache Maven / Gradle / Ivy
package com.github.chaosfirebolt.converter.api.cache;
import com.github.chaosfirebolt.converter.api.cache.storage.Computation;
import com.github.chaosfirebolt.converter.api.cache.storage.Storage;
import com.github.chaosfirebolt.converter.api.initialization.InitializationCapable;
import com.github.chaosfirebolt.converter.api.initialization.InitializationData;
import com.github.chaosfirebolt.converter.api.initialization.NoOpMapData;
import java.util.Map;
import java.util.function.Supplier;
/**
* The default cache implementation.
*
* @param key type
* @param value type
*/
public class DefaultCache implements Cache, InitializationCapable {
private final Storage storage;
private final Computation computation;
private final InitializationData
© 2015 - 2025 Weber Informatics LLC | Privacy Policy