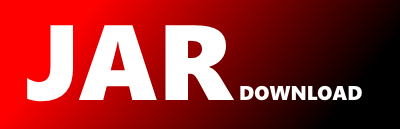
com.github.charleslzq.baiduface.client.BaiduFaceApi.kt Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of baidu-face-server Show documentation
Show all versions of baidu-face-server Show documentation
baidu face server, make request to baidu face open api for client
The newest version!
package com.github.charleslzq.baiduface.client
import com.baidu.aip.face.AipFace
import com.github.charleslzq.baiduface.client.io.*
import com.google.gson.Gson
import org.json.JSONObject
interface BaiduFaceApi {
fun detect(image: Image, options: DetectOptions = DetectOptions()): BaiduResponse
fun search(image: Image, vararg groupId: String, userId: String? = null, options: SearchOptions = SearchOptions()): BaiduResponse
fun match(vararg images: MatchReq): BaiduResponse
fun verify(vararg verifyReq: FaceVerifyReq): BaiduResponse
fun listGroup(options: PageOptions): BaiduResponse
fun addGroup(groupId: String): BaiduResponse<*>
fun deleteGroup(groupId: String): BaiduResponse<*>
fun registerUser(image: Image, userMeta: UserMeta, options: ImageOptions = ImageOptions()): BaiduResponse
fun updateUser(image: Image, userMeta: UserMeta, options: ImageOptions = ImageOptions()): BaiduResponse
fun listUser(groupId: String, options: PageOptions = PageOptions()): BaiduResponse
fun copyUser(userId: String, srcGroup: String, dstGroup: String): BaiduResponse<*>
fun getUser(groupId: String, userId: String): BaiduResponse
fun deleteUser(groupId: String, userId: String): BaiduResponse<*>
fun listFace(groupId: String, userId: String): BaiduResponse
fun deleteFace(groupId: String, userId: String, faceToken: String): BaiduResponse<*>
}
class BaiduFaceApiAdapter(
private val client: AipFace,
private val gson: Gson
) : BaiduFaceApi {
override fun detect(image: Image, options: DetectOptions) = DetectResult.fromBaidu {
options.check()
client.detect(image.data, image.type.name, options.toHashmap())
}
override fun search(image: Image, vararg groupId: String, userId: String?, options: SearchOptions) = SearchResult.fromBaidu {
options.check()
client.search(image.data, image.type.name, groupId.joinToString(","), options.withUserId(userId))
}
override fun match(vararg images: MatchReq) = MatchResult.fromBaidu {
client.match(images.map { it.toMatchRequest() })
}
override fun verify(vararg verifyReq: FaceVerifyReq) = VerifyResult.fromBaidu {
client.faceverify(verifyReq.map { it.toFaceVerifyRequest() })
}
override fun listGroup(options: PageOptions) = GroupIdList.fromBaidu {
options.check()
client.getGroupList(options.toHashmap())
}
override fun addGroup(groupId: String) = BaiduResponse.fromBaidu { client.groupAdd(groupId, hashMapOf()) }
override fun deleteGroup(groupId: String) = BaiduResponse.fromBaidu { client.groupDelete(groupId, hashMapOf()) }
override fun registerUser(image: Image, userMeta: UserMeta, options: ImageOptions) = FaceOperationResult.fromBaidu {
options.check()
client.addUser(image.data, image.type.name, userMeta.groupId, userMeta.userId, options.withUserData(userMeta))
}
override fun updateUser(image: Image, userMeta: UserMeta, options: ImageOptions) = FaceOperationResult.fromBaidu {
options.check()
client.updateUser(image.data, image.type.name, userMeta.groupId, userMeta.userId, options.withUserData(userMeta))
}
override fun listUser(groupId: String, options: PageOptions) = UserIdList.fromBaidu {
options.check()
client.getGroupUsers(groupId, options.toHashmap())
}
override fun copyUser(userId: String, srcGroup: String, dstGroup: String) = BaiduResponse.fromBaidu {
client.userCopy(userId, hashMapOf(
"src_group_id" to srcGroup,
"dst_group_id" to dstGroup
))
}
override fun getUser(groupId: String, userId: String) = UserQueryResult.fromBaidu { client.getUser(userId, groupId, hashMapOf()) }
override fun deleteUser(groupId: String, userId: String) = BaiduResponse.fromBaidu { client.deleteUser(groupId, userId, hashMapOf()) }
override fun listFace(groupId: String, userId: String) = FaceListResult.fromBaidu { client.faceGetlist(userId, groupId, hashMapOf()) }
override fun deleteFace(groupId: String, userId: String, faceToken: String) = BaiduResponse.fromBaidu { client.faceDelete(userId, groupId, faceToken, hashMapOf()) }
private fun FromJson.fromBaidu(get: () -> JSONObject) = handleException {
fromJson(gson, get)
}
private fun handleException(handler: (Throwable) -> BaiduResponse = this::defaultExceptionHandler, get: () -> BaiduResponse): BaiduResponse {
return try {
get()
} catch (throwable: Throwable) {
handler(throwable)
}
}
private fun defaultExceptionHandler(throwable: Throwable): BaiduResponse = BaiduResponse.errResponse(throwable.localizedMessage)
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy