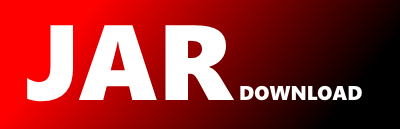
com.github.chen0040.mlp.functions.RangeScaler Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of java-ann-mlp Show documentation
Show all versions of java-ann-mlp Show documentation
Multi-Layer Perceptron with BP learning implemented in Java
package com.github.chen0040.mlp.functions;
import lombok.Getter;
import lombok.Setter;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
/**
* Created by xschen on 30/5/2017.
*/
@Getter
@Setter
public class RangeScaler implements Cloneable {
private final Map minValue = new HashMap<>();
private final Map maxValue = new HashMap<>();
public RangeScaler(List targets) {
for(int i = 0; i < targets.size(); ++i){
double[] values = targets.get(i);
for(int j=0; j < values.length; ++j) {
minValue.put(j, Math.min(minValue.getOrDefault(j, Double.MAX_VALUE), values[j]));
maxValue.put(j, Math.max(maxValue.getOrDefault(j, Double.NEGATIVE_INFINITY), values[j]));
}
}
}
@Override
public Object clone() throws CloneNotSupportedException {
RangeScaler obj = (RangeScaler)super.clone();
obj.getMaxValue().putAll(maxValue);
obj.getMinValue().putAll(minValue);
return obj;
}
public double[] standardize(double[] target) {
double[] result = new double[target.length];
for(int i=0; i < result.length; ++i){
result[i] = (target[i] - minValue.get(i)) / (maxValue.get(i) - minValue.get(i));
}
return result;
}
public double[] revert(double[] target) {
double[] result = new double[target.length];
for(int i=0; i < result.length; ++i){
result[i] = minValue.get(i) + target[i] * (maxValue.get(i) - minValue.get(i));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy