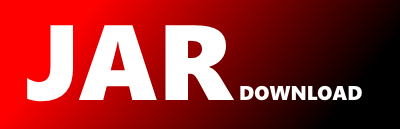
com.github.chengpohi.parser.ELKInstructionParser.scala Maven / Gradle / Ivy
The newest version!
package com.github.chengpohi.parser
import com.github.chengpohi.api.dsl.{Definition, ExtractDefinition}
import com.github.chengpohi.collection.JsonCollection
import com.github.chengpohi.collection.JsonCollection.{Str, Val}
import fastparse.noApi._
/**
* elasticservice
* Created by chengpohi on 1/18/16.
*/
class ELKInstructionParser(val interceptFunction: InterceptFunction) extends CollectionParser {
import WhitespaceApi._
val help = P(alphaChars.rep(1).! ~ "?")
.map(JsonCollection.Str)
.map(s => ("help", Some(interceptFunction.help), Seq(s)))
val health = P("health").map(s => Instruction("health", interceptFunction.health, Seq(Str(""))))
val shutdown = P("shutdown").map(s => Instruction("shutdown", interceptFunction.shutdown, Seq(Str(""))))
val count = P("count" ~/ ioParser).map(c => Instruction("count", interceptFunction.count, c))
//memory, jvm, nodes, cpu etc
val clusterStats = P("cluster stats").map(s => Instruction("clusterStats", interceptFunction.clusterStats, Seq()))
//indices, aliases, restore, snapshots, routing nodes etc
val clusterState = P("cluster state").map(s => Instruction("clusterState", interceptFunction.getIndices, Seq()))
val indicesStats = P("indices stats").map(s => Instruction("indiciesStats", interceptFunction.indicesStats, Seq()))
val nodeStats = P("node stats").map(s => Instruction("nodeStats", interceptFunction.nodeStats, Seq()))
val clusterSettings = P("cluster settings").map(s => Instruction("clusterSettings", interceptFunction.clusterSettings, Seq()))
val nodeSettings = P("node settings").map(s => Instruction("nodeSettings", interceptFunction.nodeSettings, Seq()))
val indexSettings = P(ioParser ~ "settings").map(s => Instruction("nodeSettings", interceptFunction.indexSettings, s))
val pendingTasks = P("pending tasks").map(s => Instruction("pendingTasks", interceptFunction.pendingTasks, Seq()))
val waitForStatus = P("wait for status" ~ ioParser).map(s => Instruction("pendingTasks", interceptFunction.waitForStatus, s))
val deleteDoc = P("delete" ~ "from" ~/ strOrVar ~ "/" ~/ strOrVar ~ "id" ~ strOrVar)
.map(c => Instruction("delete", interceptFunction.deleteDoc, Seq(c._1, c._2, c._3)))
val deleteIndex = P("delete" ~ "index" ~/ strOrVar).map(c => Instruction("delete", interceptFunction.deleteIndex, Seq(c)))
val termQuery = P("term" ~ "query" ~/ ioParser).map(c => Instruction("termQuery", interceptFunction.query, c))
val joinSearch = P("join" ~ strOrVar ~ "/" ~ strOrVar ~ "by" ~ strOrVar)
.map(c => Instruction("joinQuery", interceptFunction.joinQuery, Seq(c._1, c._2, c._3)))
val matchQuery = P("match" ~/ jsonExpr)
.map(c => Instruction("matchQuery", interceptFunction.matchQuery, Seq(c)))
val search: P[Instruction] = P("search" ~ "in" ~/ strOrVar ~ ("/" ~ strOrVar ~ (matchQuery | joinSearch).?).?)
.map(c => c._2 match {
case None => Instruction("query", interceptFunction.query, Seq(c._1))
case Some((f, None)) => Instruction("query", interceptFunction.query, Seq(c._1, f))
case Some((f, Some(t))) => Instruction(t.name, t.f, Seq(c._1, f) ++ t.params)
})
val reindex = P("reindex" ~ "into" ~ strOrVar ~ "/" ~/ strOrVar ~ "from" ~/ strOrVar ~ "fields" ~/ jsonExpr)
.map(c => Instruction("reindex", interceptFunction.reindexIndex, Seq(c._1, c._2, c._3, c._4)))
val index = P("index" ~ "into" ~/ strOrVar ~ "/" ~ strOrVar ~/ "fields" ~ jsonExpr ~ ("id" ~ strOrVar).?)
.map(c => Instruction("index", interceptFunction.createDoc, Seq(c._1, c._2, c._3) ++ c._4.toSeq))
val bulkIndex = P("bulk index" ~/ ioParser).map(c => Instruction("bulkIndex", interceptFunction.bulkIndex, c))
val updateMapping = P("update mapping" ~/ ioParser).map(c => Instruction("umapping", interceptFunction.updateMapping, c))
val update = P("update" ~ "on" ~/ strOrVar ~ "/" ~ strOrVar ~ "fields" ~/ jsonExpr ~ ("id" ~ strOrVar).?)
.map(c => {
c._4 match {
case None => Instruction("update", interceptFunction.bulkUpdateDoc, Seq(c._1, c._2, c._3))
case Some(id) => Instruction("update", interceptFunction.updateDoc, Seq(c._1, c._2, c._3) ++ c._4.toSeq)
}
})
val createIndex = P("create" ~ "index" ~/ strOrVar).map(c => Instruction("createIndex", interceptFunction.createIndex, Seq(c)))
val getMapping = P(strOrVar ~ "mapping").map(c => Instruction("getMapping", interceptFunction.getMapping, Seq(c)))
val analysis = P("analysis" ~/ strOrVar ~/ "by" ~/ strOrVar).map(c => Instruction("analysis", interceptFunction.analysisText, Seq(c._1, c._2)))
val createAnalyzer = P("create analyzer" ~/ ioParser).map(c => Instruction("createAnalyzer", interceptFunction.createAnalyzer, c))
val getDocById = P("get" ~ "from" ~/ strOrVar ~ "/" ~/ strOrVar ~ "id" ~/ strOrVar)
.map(c => Instruction("getDocById", interceptFunction.getDocById, Seq(c._1, c._2, c._3)))
val mapping = P("mapping" ~/ ioParser).map(c => Instruction("mapping", interceptFunction.mapping, c))
val avgAggs = P("avg" ~/ strOrVar).map(c => Instruction("avgAggs", interceptFunction.aggsCount, Seq(c)))
val termsAggs = P("term" ~/ strOrVar).map(c => Instruction("termAggs", interceptFunction.aggsTerm, Seq(c)))
val histAggs = P("hist" ~/ strOrVar ~ "interval" ~/ strOrVar ~/ "field" ~ strOrVar)
.map(c => Instruction("histAggs", interceptFunction.histAggs, Seq(c._1, c._2, c._3)))
val aggs = P("aggs in" ~/ strOrVar ~ "/" ~ strOrVar ~/ (avgAggs | termsAggs | histAggs))
.map(c => Instruction(c._3.name, c._3.f, Seq(c._1, c._2) ++ c._3.params))
val alias = P("alias" ~/ ioParser).map(c => Instruction("alias", interceptFunction.alias, c))
val createRepository = P("create repository" ~/ ioParser).map(c => Instruction("createRepository", interceptFunction.createRepository, c))
val createSnapshot = P("create snapshot " ~/ ioParser).map(c => Instruction("createSnapshot", interceptFunction.createSnapshot, c))
val deleteSnapshot = P("delete snapshot " ~/ ioParser).map(c => Instruction("deleteSnapshot", interceptFunction.deleteSnapshot, c))
val getSnapshot = P("get snapshot " ~/ ioParser).map(c => Instruction("getSnapshot", interceptFunction.getSnapshot, c))
val restoreSnapshot = P("restore snapshot " ~/ ioParser).map(c => Instruction("restoreSnapshot", interceptFunction.restoreSnapshot, c))
val closeIndex = P("close index" ~/ ioParser).map(c => Instruction("closeIndex", interceptFunction.closeIndex, c))
val openIndex = P("open index" ~/ ioParser).map(c => Instruction("openIndex", interceptFunction.openIndex, c))
val dumpIndex = P("dump index" ~/ strOrVar ~/ ">" ~/ strChars.rep(1).!)
.map(c => Instruction("dumpIndex", interceptFunction.dumpIndex, Seq(c._1, JsonCollection.Str(c._2))))
val extractJSON = P("\\\\" ~ strOrVar).map(c => ("extract", c.value))
//val beauty = P("beauty").map(c => ("beauty", beautyJson))
val instrument: P[Instruction] = P((health | shutdown | clusterStats | indicesStats | nodeStats | pendingTasks | waitForStatus
| clusterSettings | nodeSettings | indexSettings | clusterState
| restoreSnapshot | deleteSnapshot | createSnapshot | getSnapshot | createRepository
| deleteDoc | deleteIndex
| search | termQuery | getDocById
| reindex | index | bulkIndex | createIndex | closeIndex | openIndex | dumpIndex
| updateMapping | update | analysis | aggs | createAnalyzer
| getMapping | mapping
| alias | count) ~ extractJSON.?).map(t => {
t._2 match {
case Some((name, s)) => {
val f = buildExtractDefinition(t._1.f, s)
t._1.copy(f = f)
}
case None => t._1
}
})
case class Instruction(name: String, f: Seq[Val] => Definition[_], params: Seq[Val])
def buildExtractDefinition(f: Seq[Val] => Definition[_], path: String): Seq[Val] => ExtractDefinition = {
val f2: Definition[_] => ExtractDefinition = ExtractDefinition(_, path)
f andThen f2
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy