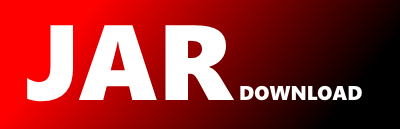
org.modelmapper.module.jsr310.ToTemporalConverter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of modelmapper-module-jsr310 Show documentation
Show all versions of modelmapper-module-jsr310 Show documentation
ModelMapper Module for JSR310
package org.modelmapper.module.jsr310;
import java.time.Instant;
import java.time.LocalDate;
import java.time.LocalDateTime;
import java.time.format.DateTimeFormatter;
import java.time.temporal.Temporal;
import java.util.Calendar;
import java.util.Date;
import org.modelmapper.Converter;
import org.modelmapper.internal.Errors;
import org.modelmapper.spi.ConditionalConverter;
import org.modelmapper.spi.MappingContext;
/**
* Converts {@link Object} to {@link Temporal}
*
* @author Chun Han Hsiao
*/
public class ToTemporalConverter implements ConditionalConverter
© 2015 - 2025 Weber Informatics LLC | Privacy Policy