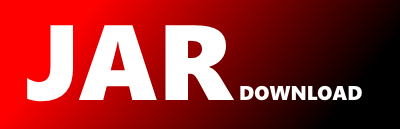
se.claremont.taf.websupport.elementidentification.By Maven / Gradle / Ivy
The newest version!
package se.claremont.taf.websupport.elementidentification;
import org.openqa.selenium.WebElement;
import java.io.Serializable;
import java.util.ArrayList;
import java.util.List;
///
/// The TAF By class extends the Selenium one by chaining different
/// Selenium alternatives together.
/// Partial texts are also introduced for all element types, and matching
/// of texts by regular expressions also is introduced, as well as using
/// a parent element to limit searches to descendants.
/// Be aware that all criterias can be combined except the CSS one, or the
/// XPath one. If any of the latter is chosen this will always be run as is,
/// without parsing the rest of the criterias. This is because it is not
/// possible to combine xpath and CSS as search attributes, and the other
/// criteria is used to build an xpath expression when trying to identify
/// an element.
///
@SuppressWarnings("unused")
public class By implements Serializable{
final List conditions;
By() {
conditions = new ArrayList<>();
}
public int getConditionCount(){
return conditions.size();
}
///
/// Filters out elements that do not have this class stated. Class names do not need to be in the same order as on page.
///
/// Class name(s) of element to search for
///
public static By classContains(String className) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "classcontains", className));
return s;
}
///
/// Filters out all element not having the exact class string as entered below.
///
/// Class name(s) of element to search for
///
public static By className(String className) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "class", className));
return s;
}
///
/// Identifying elements by xPath expression. If several elements matches the xPath
/// the first will be retrieved, but this will be logged to the test case log.
///
/// XPath expression
///
public static By xpath(String xPath) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.XPath, xPath));
return s;
}
///
/// Sometimes you want to limit your search to the descendants of a parent element. If such an element is given here, it will be the top node for the search.
///
/// The root node for the search.
///
public static By beingDescendantOf(WebElement webElement) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.ByAncestor, webElement));
return s;
}
///
/// Looking for elements with this exact text as InnerHTML
///
/// Exact text
///
public static By exactText(String text) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.ExactText, text));
return s;
}
///
/// For language sensitive automations sometimes different texts represent the
/// element in different contexts. With this method you can state as many
/// alternative texts as preffered.
///
/// Any number of strings
///
public static By exactTextMatchOfAnyOfTheStrings(String... textStrings) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.ExactText, (Object[]) textStrings));
return s;
}
///
/// For language sensitive automations sometimes different texts represent the
/// element in different contexts. With this method you can state as many
/// alternative texts as preffered.
///
/// Any number of strings
///
public static By textContainsAnyOfTheStrings(String... textStrings) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.TextContains, (Object[]) textStrings));
return s;
}
///
/// Identifies an element given its id attribute.
///
/// The id of the element to identify
///
public static By id(String id) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "id", id));
return s;
}
///
/// Identifies an element given its name attribute.
///
/// The name of the element to identify
///
public static By name(String name) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.AttributeValue, name));
return s;
}
///
/// Limits the search scope to a certain type of element, based on the html tag names.
///
/// HTML tag name
///
public static By tagName(String tagName) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.ControlType, tagName));
return s;
}
///
/// If several elements are identified with the conditions applied, this could be used
/// to state wich one you want to interact with.
///
/// Ordinal number, given the DOM tree parsing algorithm used.
///
public static By ordinalNumber(int ordinalNumber) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.OrdinalNumber, ordinalNumber));
return s;
}
///
/// Identifies elements given the text matching the regular expression pattern.
///
/// Regular expression pattern
///
public static By textIsRegexMatch(String regularExpressionPattern) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.TextRegex, regularExpressionPattern));
return s;
}
///
/// Identifies elements containing the specified String as InnerHTML.
///
/// Text to find within the element.
///
public static By textContainsString(String text) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.TextContains, text));
return s;
}
///
/// Identifies elements based on their properties, e.g.
///
/// a html element described by "input class='myclass' type='checkbox' value='first name'"
/// has property name 'type' for property value 'checkbox'.
///
///
///
///
public static By attributeValue(String attributeName, String attributeValue) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.AttributeValue, attributeName, attributeValue));
return s;
}
///
/// Identifies element with the same syntax as CSS locators in the HTML style section
///
///
///
public static By cssSelector(String cssLocator) {
By s = new By();
s.conditions.add(new SearchCondition(SearchConditionType.CssLocator, cssLocator));
return s;
}
///
/// Refines selection of element by adding class name or class names. Class names
/// filter treates class names regardless of their order.
///
/// The class name or class names to match with
///
public By andByClassContains(String className) {
conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "classcontains", className));
return this;
}
///
/// Refines element identification by exact class string match.
///
/// The class string to match
///
public By andByClass(String className) {
conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "class", className));
return this;
}
///
/// Selecting an element based on its ordinal value from within a search scope. First element is element 1.
/// Ordinal numbers are always run last, when other filter mechanisms have already been applied.
///
/// Element number. First element is element 1.
///
public By andByOrdinalNumber(int ordinalNumber) {
conditions.add(new SearchCondition(SearchConditionType.OrdinalNumber, ordinalNumber));
return this;
}
///
/// Limiting search scope to elements with a certain HTML tag.
///
/// Any of the official HTML tags, or your own ones.
///
public By andByTagName(String tagName) {
conditions.add(new SearchCondition(SearchConditionType.ControlType, tagName));
return this;
}
///
/// Limiting search scope to elements with a specific text, based on exact matching.
///
///
///
public By andByExactText(String exactText) {
conditions.add(new SearchCondition(SearchConditionType.ExactText, exactText));
return this;
}
///
/// Limiting search scope to elements with a secific text, based on regular expression pattern.
///
///
///
public By andByTextIsRegexMatch(String regexPattern) {
conditions.add(new SearchCondition(SearchConditionType.TextRegex, regexPattern));
return this;
}
///
/// Limiting search scope to elements containing a specific text.
///
///
///
public By andByTextContainsString(String textContained) {
conditions.add(new SearchCondition(SearchConditionType.TextContains, textContained));
return this;
}
///
/// Limiting search scope to elements with a specific name as an attribute.
///
///
///
public By andByElementName(String name) {
conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "name", name));
return this;
}
///
/// Limiting search scope to elements with a specific id as an attribute.
///
///
///
public By andById(String id) {
conditions.add(new SearchCondition(SearchConditionType.AttributeValue, "id", id));
return this;
}
///
/// Limiting search scope for specific attributes.
///
///
///
///
public By andByAttributeValue(String attributeName, String attributeValue) {
conditions.add(new SearchCondition(SearchConditionType.AttributeValue, attributeName, attributeValue));
return this;
}
///
/// Limits search scope to the descendantso of a specific element, known as the ancestor.
///
///
///
public By andByBeingDescendantOf(WebElement ancestor) {
conditions.add(new SearchCondition(SearchConditionType.ByAncestor, ancestor));
return this;
}
///
/// For language sensitive automations sometimes different texts represent the
/// element in different contexts. With this method you can state as many
/// alternative texts as preffered.
///
/// Any number of strings
///
public By andByTextBeingExactlyAnyOfTheStrings(String... textStrings) {
conditions.add(new SearchCondition(SearchConditionType.ExactText, (Object[]) textStrings));
return this;
}
///
/// For language sensitive automations sometimes different texts represent the
/// element in different contexts. With this method you can state as many
/// alternative texts as preffered.
///
/// Any number of strings
///
public By andByTextContainingAnyOfTheStrings(String... textStrings) {
conditions.add(new SearchCondition(SearchConditionType.TextContains, (Object[]) textStrings));
return this;
}
///
/// Finding the element based on specific explicit xPath. If several elements
/// are matched, an ordinal number can be applied.
///
///
///
public By andByXPath(String xPath) {
conditions.add(new SearchCondition(SearchConditionType.XPath, xPath));
return this;
}
@Override
public String toString() {
String returnString = "[By:" + System.lineSeparator();
for (SearchCondition sc : conditions) {
returnString += " " + sc.toString() + System.lineSeparator();
}
return returnString + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy