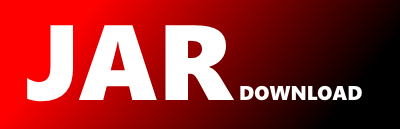
control.ControlMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/control/controlmessage.proto
package control;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoEnum;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.ENUM;
import static com.squareup.wire.Message.Datatype.INT64;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / ControlMessage wraps a control command and adds metadata.
*/
public final class ControlMessage extends Message {
public static final String DEFAULT_ORIGIN = "";
public static final Long DEFAULT_TIMESTAMP = 0L;
public static final ControlType DEFAULT_CONTROLTYPE = ControlType.HeartbeatRequest;
@ProtoField(tag = 1, type = STRING, label = REQUIRED)
public final String origin;
/**
* / Unique description of the origin of this event.
*/
@ProtoField(tag = 2, label = REQUIRED)
public final UUID identifier;
/**
* / A unique identifier for this control
*/
@ProtoField(tag = 3, type = INT64, label = REQUIRED)
public final Long timestamp;
/**
* / UNIX timestamp (in nanoseconds) event was wrapped in this ControlMessage.
*/
@ProtoField(tag = 4, type = ENUM, label = REQUIRED)
public final ControlType controlType;
/**
* / Type of wrapped control. Only the optional field corresponding to the value of ControlType should be set.
*/
@ProtoField(tag = 5)
public final HeartbeatRequest heartbeatRequest;
public ControlMessage(String origin, UUID identifier, Long timestamp, ControlType controlType, HeartbeatRequest heartbeatRequest) {
this.origin = origin;
this.identifier = identifier;
this.timestamp = timestamp;
this.controlType = controlType;
this.heartbeatRequest = heartbeatRequest;
}
private ControlMessage(Builder builder) {
this(builder.origin, builder.identifier, builder.timestamp, builder.controlType, builder.heartbeatRequest);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof ControlMessage)) return false;
ControlMessage o = (ControlMessage) other;
return equals(origin, o.origin)
&& equals(identifier, o.identifier)
&& equals(timestamp, o.timestamp)
&& equals(controlType, o.controlType)
&& equals(heartbeatRequest, o.heartbeatRequest);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = origin != null ? origin.hashCode() : 0;
result = result * 37 + (identifier != null ? identifier.hashCode() : 0);
result = result * 37 + (timestamp != null ? timestamp.hashCode() : 0);
result = result * 37 + (controlType != null ? controlType.hashCode() : 0);
result = result * 37 + (heartbeatRequest != null ? heartbeatRequest.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public String origin;
public UUID identifier;
public Long timestamp;
public ControlType controlType;
public HeartbeatRequest heartbeatRequest;
public Builder() {
}
public Builder(ControlMessage message) {
super(message);
if (message == null) return;
this.origin = message.origin;
this.identifier = message.identifier;
this.timestamp = message.timestamp;
this.controlType = message.controlType;
this.heartbeatRequest = message.heartbeatRequest;
}
public Builder origin(String origin) {
this.origin = origin;
return this;
}
/**
* / Unique description of the origin of this event.
*/
public Builder identifier(UUID identifier) {
this.identifier = identifier;
return this;
}
/**
* / A unique identifier for this control
*/
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / UNIX timestamp (in nanoseconds) event was wrapped in this ControlMessage.
*/
public Builder controlType(ControlType controlType) {
this.controlType = controlType;
return this;
}
/**
* / Type of wrapped control. Only the optional field corresponding to the value of ControlType should be set.
*/
public Builder heartbeatRequest(HeartbeatRequest heartbeatRequest) {
this.heartbeatRequest = heartbeatRequest;
return this;
}
@Override
public ControlMessage build() {
checkRequiredFields();
return new ControlMessage(this);
}
}
public enum ControlType
implements ProtoEnum {
HeartbeatRequest(1);
private final int value;
private ControlType(int value) {
this.value = value;
}
@Override
public int getValue() {
return value;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy