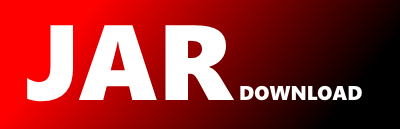
events.ContainerMetric Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/metric.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.DOUBLE;
import static com.squareup.wire.Message.Datatype.INT32;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Datatype.UINT64;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / A ContainerMetric records resource usage of an app in a container.
*/
public final class ContainerMetric extends Message {
public static final String DEFAULT_APPLICATIONID = "";
public static final Integer DEFAULT_INSTANCEINDEX = 0;
public static final Double DEFAULT_CPUPERCENTAGE = 0D;
public static final Long DEFAULT_MEMORYBYTES = 0L;
public static final Long DEFAULT_DISKBYTES = 0L;
@ProtoField(tag = 1, type = STRING, label = REQUIRED)
public final String applicationId;
/**
* / ID of the contained application.
*/
@ProtoField(tag = 2, type = INT32, label = REQUIRED)
public final Integer instanceIndex;
/**
* / Instance index of the contained application. (This, with applicationId, should uniquely identify a container.)
*/
@ProtoField(tag = 3, type = DOUBLE, label = REQUIRED)
public final Double cpuPercentage;
/**
* / CPU used, on a scale of 0 to 100.
*/
@ProtoField(tag = 4, type = UINT64, label = REQUIRED)
public final Long memoryBytes;
/**
* / Bytes of memory used.
*/
@ProtoField(tag = 5, type = UINT64, label = REQUIRED)
public final Long diskBytes;
public ContainerMetric(String applicationId, Integer instanceIndex, Double cpuPercentage, Long memoryBytes, Long diskBytes) {
this.applicationId = applicationId;
this.instanceIndex = instanceIndex;
this.cpuPercentage = cpuPercentage;
this.memoryBytes = memoryBytes;
this.diskBytes = diskBytes;
}
private ContainerMetric(Builder builder) {
this(builder.applicationId, builder.instanceIndex, builder.cpuPercentage, builder.memoryBytes, builder.diskBytes);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof ContainerMetric)) return false;
ContainerMetric o = (ContainerMetric) other;
return equals(applicationId, o.applicationId)
&& equals(instanceIndex, o.instanceIndex)
&& equals(cpuPercentage, o.cpuPercentage)
&& equals(memoryBytes, o.memoryBytes)
&& equals(diskBytes, o.diskBytes);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = applicationId != null ? applicationId.hashCode() : 0;
result = result * 37 + (instanceIndex != null ? instanceIndex.hashCode() : 0);
result = result * 37 + (cpuPercentage != null ? cpuPercentage.hashCode() : 0);
result = result * 37 + (memoryBytes != null ? memoryBytes.hashCode() : 0);
result = result * 37 + (diskBytes != null ? diskBytes.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public String applicationId;
public Integer instanceIndex;
public Double cpuPercentage;
public Long memoryBytes;
public Long diskBytes;
public Builder() {
}
public Builder(ContainerMetric message) {
super(message);
if (message == null) return;
this.applicationId = message.applicationId;
this.instanceIndex = message.instanceIndex;
this.cpuPercentage = message.cpuPercentage;
this.memoryBytes = message.memoryBytes;
this.diskBytes = message.diskBytes;
}
public Builder applicationId(String applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* / ID of the contained application.
*/
public Builder instanceIndex(Integer instanceIndex) {
this.instanceIndex = instanceIndex;
return this;
}
/**
* / Instance index of the contained application. (This, with applicationId, should uniquely identify a container.)
*/
public Builder cpuPercentage(Double cpuPercentage) {
this.cpuPercentage = cpuPercentage;
return this;
}
/**
* / CPU used, on a scale of 0 to 100.
*/
public Builder memoryBytes(Long memoryBytes) {
this.memoryBytes = memoryBytes;
return this;
}
/**
* / Bytes of memory used.
*/
public Builder diskBytes(Long diskBytes) {
this.diskBytes = diskBytes;
return this;
}
@Override
public ContainerMetric build() {
checkRequiredFields();
return new ContainerMetric(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy