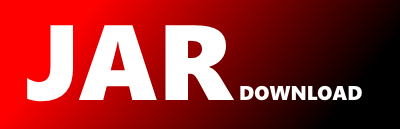
events.Envelope Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/envelope.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoEnum;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.ENUM;
import static com.squareup.wire.Message.Datatype.INT64;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / Envelope wraps an Event and adds metadata.
*/
public final class Envelope extends Message {
public static final String DEFAULT_ORIGIN = "";
public static final EventType DEFAULT_EVENTTYPE = EventType.Heartbeat;
public static final Long DEFAULT_TIMESTAMP = 0L;
@ProtoField(tag = 1, type = STRING, label = REQUIRED)
public final String origin;
/**
* / Unique description of the origin of this event.
*/
@ProtoField(tag = 2, type = ENUM, label = REQUIRED)
public final EventType eventType;
/**
* / Type of wrapped event. Only the optional field corresponding to the value of eventType should be set.
*/
@ProtoField(tag = 6, type = INT64)
public final Long timestamp;
/**
* / UNIX timestamp (in nanoseconds) event was wrapped in this Envelope.
*/
@ProtoField(tag = 3)
public final Heartbeat heartbeat;
@ProtoField(tag = 4)
public final HttpStart httpStart;
@ProtoField(tag = 5)
public final HttpStop httpStop;
@ProtoField(tag = 7)
public final HttpStartStop httpStartStop;
@ProtoField(tag = 8)
public final LogMessage logMessage;
@ProtoField(tag = 9)
public final ValueMetric valueMetric;
@ProtoField(tag = 10)
public final CounterEvent counterEvent;
@ProtoField(tag = 11)
public final Error error;
@ProtoField(tag = 12)
public final ContainerMetric containerMetric;
public Envelope(String origin, EventType eventType, Long timestamp, Heartbeat heartbeat, HttpStart httpStart, HttpStop httpStop, HttpStartStop httpStartStop, LogMessage logMessage, ValueMetric valueMetric, CounterEvent counterEvent, Error error, ContainerMetric containerMetric) {
this.origin = origin;
this.eventType = eventType;
this.timestamp = timestamp;
this.heartbeat = heartbeat;
this.httpStart = httpStart;
this.httpStop = httpStop;
this.httpStartStop = httpStartStop;
this.logMessage = logMessage;
this.valueMetric = valueMetric;
this.counterEvent = counterEvent;
this.error = error;
this.containerMetric = containerMetric;
}
private Envelope(Builder builder) {
this(builder.origin, builder.eventType, builder.timestamp, builder.heartbeat, builder.httpStart, builder.httpStop, builder.httpStartStop, builder.logMessage, builder.valueMetric, builder.counterEvent, builder.error, builder.containerMetric);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof Envelope)) return false;
Envelope o = (Envelope) other;
return equals(origin, o.origin)
&& equals(eventType, o.eventType)
&& equals(timestamp, o.timestamp)
&& equals(heartbeat, o.heartbeat)
&& equals(httpStart, o.httpStart)
&& equals(httpStop, o.httpStop)
&& equals(httpStartStop, o.httpStartStop)
&& equals(logMessage, o.logMessage)
&& equals(valueMetric, o.valueMetric)
&& equals(counterEvent, o.counterEvent)
&& equals(error, o.error)
&& equals(containerMetric, o.containerMetric);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = origin != null ? origin.hashCode() : 0;
result = result * 37 + (eventType != null ? eventType.hashCode() : 0);
result = result * 37 + (timestamp != null ? timestamp.hashCode() : 0);
result = result * 37 + (heartbeat != null ? heartbeat.hashCode() : 0);
result = result * 37 + (httpStart != null ? httpStart.hashCode() : 0);
result = result * 37 + (httpStop != null ? httpStop.hashCode() : 0);
result = result * 37 + (httpStartStop != null ? httpStartStop.hashCode() : 0);
result = result * 37 + (logMessage != null ? logMessage.hashCode() : 0);
result = result * 37 + (valueMetric != null ? valueMetric.hashCode() : 0);
result = result * 37 + (counterEvent != null ? counterEvent.hashCode() : 0);
result = result * 37 + (error != null ? error.hashCode() : 0);
result = result * 37 + (containerMetric != null ? containerMetric.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public String origin;
public EventType eventType;
public Long timestamp;
public Heartbeat heartbeat;
public HttpStart httpStart;
public HttpStop httpStop;
public HttpStartStop httpStartStop;
public LogMessage logMessage;
public ValueMetric valueMetric;
public CounterEvent counterEvent;
public Error error;
public ContainerMetric containerMetric;
public Builder() {
}
public Builder(Envelope message) {
super(message);
if (message == null) return;
this.origin = message.origin;
this.eventType = message.eventType;
this.timestamp = message.timestamp;
this.heartbeat = message.heartbeat;
this.httpStart = message.httpStart;
this.httpStop = message.httpStop;
this.httpStartStop = message.httpStartStop;
this.logMessage = message.logMessage;
this.valueMetric = message.valueMetric;
this.counterEvent = message.counterEvent;
this.error = message.error;
this.containerMetric = message.containerMetric;
}
public Builder origin(String origin) {
this.origin = origin;
return this;
}
/**
* / Unique description of the origin of this event.
*/
public Builder eventType(EventType eventType) {
this.eventType = eventType;
return this;
}
/**
* / Type of wrapped event. Only the optional field corresponding to the value of eventType should be set.
*/
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / UNIX timestamp (in nanoseconds) event was wrapped in this Envelope.
*/
public Builder heartbeat(Heartbeat heartbeat) {
this.heartbeat = heartbeat;
return this;
}
public Builder httpStart(HttpStart httpStart) {
this.httpStart = httpStart;
return this;
}
public Builder httpStop(HttpStop httpStop) {
this.httpStop = httpStop;
return this;
}
public Builder httpStartStop(HttpStartStop httpStartStop) {
this.httpStartStop = httpStartStop;
return this;
}
public Builder logMessage(LogMessage logMessage) {
this.logMessage = logMessage;
return this;
}
public Builder valueMetric(ValueMetric valueMetric) {
this.valueMetric = valueMetric;
return this;
}
public Builder counterEvent(CounterEvent counterEvent) {
this.counterEvent = counterEvent;
return this;
}
public Builder error(Error error) {
this.error = error;
return this;
}
public Builder containerMetric(ContainerMetric containerMetric) {
this.containerMetric = containerMetric;
return this;
}
@Override
public Envelope build() {
checkRequiredFields();
return new Envelope(this);
}
}
public enum EventType
implements ProtoEnum {
Heartbeat(1),
HttpStart(2),
HttpStop(3),
HttpStartStop(4),
LogMessage(5),
ValueMetric(6),
CounterEvent(7),
Error(8),
ContainerMetric(9);
private final int value;
private EventType(int value) {
this.value = value;
}
@Override
public int getValue() {
return value;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy