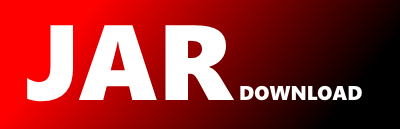
events.Heartbeat Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/heartbeat.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.UINT64;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / A Heartbeat event both indicates liveness of the emitter, and communicates counts of events processed.
*/
public final class Heartbeat extends Message {
public static final Long DEFAULT_SENTCOUNT = 0L;
public static final Long DEFAULT_RECEIVEDCOUNT = 0L;
public static final Long DEFAULT_ERRORCOUNT = 0L;
@ProtoField(tag = 1, type = UINT64, label = REQUIRED)
public final Long sentCount;
/**
* / Number of events sent by this emitter.
*/
@ProtoField(tag = 2, type = UINT64, label = REQUIRED)
public final Long receivedCount;
/**
* / Number of events received by this emitter from the host process.
*/
@ProtoField(tag = 3, type = UINT64, label = REQUIRED)
public final Long errorCount;
/**
* / Number of errors encountered while sending.
*/
@ProtoField(tag = 4)
public final UUID controlMessageIdentifier;
public Heartbeat(Long sentCount, Long receivedCount, Long errorCount, UUID controlMessageIdentifier) {
this.sentCount = sentCount;
this.receivedCount = receivedCount;
this.errorCount = errorCount;
this.controlMessageIdentifier = controlMessageIdentifier;
}
private Heartbeat(Builder builder) {
this(builder.sentCount, builder.receivedCount, builder.errorCount, builder.controlMessageIdentifier);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof Heartbeat)) return false;
Heartbeat o = (Heartbeat) other;
return equals(sentCount, o.sentCount)
&& equals(receivedCount, o.receivedCount)
&& equals(errorCount, o.errorCount)
&& equals(controlMessageIdentifier, o.controlMessageIdentifier);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = sentCount != null ? sentCount.hashCode() : 0;
result = result * 37 + (receivedCount != null ? receivedCount.hashCode() : 0);
result = result * 37 + (errorCount != null ? errorCount.hashCode() : 0);
result = result * 37 + (controlMessageIdentifier != null ? controlMessageIdentifier.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public Long sentCount;
public Long receivedCount;
public Long errorCount;
public UUID controlMessageIdentifier;
public Builder() {
}
public Builder(Heartbeat message) {
super(message);
if (message == null) return;
this.sentCount = message.sentCount;
this.receivedCount = message.receivedCount;
this.errorCount = message.errorCount;
this.controlMessageIdentifier = message.controlMessageIdentifier;
}
public Builder sentCount(Long sentCount) {
this.sentCount = sentCount;
return this;
}
/**
* / Number of events sent by this emitter.
*/
public Builder receivedCount(Long receivedCount) {
this.receivedCount = receivedCount;
return this;
}
/**
* / Number of events received by this emitter from the host process.
*/
public Builder errorCount(Long errorCount) {
this.errorCount = errorCount;
return this;
}
/**
* / Number of errors encountered while sending.
*/
public Builder controlMessageIdentifier(UUID controlMessageIdentifier) {
this.controlMessageIdentifier = controlMessageIdentifier;
return this;
}
@Override
public Heartbeat build() {
checkRequiredFields();
return new Heartbeat(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy