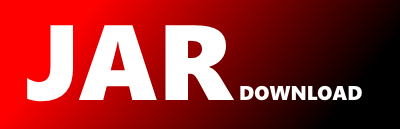
events.HttpStart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/http.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.ENUM;
import static com.squareup.wire.Message.Datatype.INT32;
import static com.squareup.wire.Message.Datatype.INT64;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / An HttpStart event is emitted when a client sends a request (or immediately when a server receives the request).
*/
public final class HttpStart extends Message {
public static final Long DEFAULT_TIMESTAMP = 0L;
public static final PeerType DEFAULT_PEERTYPE = PeerType.Client;
public static final Method DEFAULT_METHOD = Method.GET;
public static final String DEFAULT_URI = "";
public static final String DEFAULT_REMOTEADDRESS = "";
public static final String DEFAULT_USERAGENT = "";
public static final Integer DEFAULT_INSTANCEINDEX = 0;
public static final String DEFAULT_INSTANCEID = "";
@ProtoField(tag = 1, type = INT64, label = REQUIRED)
public final Long timestamp;
/**
* / UNIX timestamp (in nanoseconds) when the request was sent (by a client) or received (by a server).
*/
@ProtoField(tag = 2, label = REQUIRED)
public final UUID requestId;
/**
* / ID for tracking lifecycle of request.
*/
@ProtoField(tag = 3, type = ENUM, label = REQUIRED)
public final PeerType peerType;
/**
* / Role of the emitting process in the request cycle.
*/
@ProtoField(tag = 4, type = ENUM, label = REQUIRED)
public final Method method;
/**
* / Method of the request.
*/
@ProtoField(tag = 5, type = STRING, label = REQUIRED)
public final String uri;
/**
* / Destination of the request.
*/
@ProtoField(tag = 6, type = STRING, label = REQUIRED)
public final String remoteAddress;
/**
* / Remote address of the request. (For a server, this should be the origin of the request.)
*/
@ProtoField(tag = 7, type = STRING, label = REQUIRED)
public final String userAgent;
/**
* / Contents of the UserAgent header on the request.
*/
@ProtoField(tag = 8)
public final UUID parentRequestId;
/**
* / If this request was made in order to service an incoming request, this field should track the ID of the parent.
*/
@ProtoField(tag = 9)
public final UUID applicationId;
/**
* / If this request was made in relation to an appliciation, this field should track that application's ID.
*/
@ProtoField(tag = 10, type = INT32)
public final Integer instanceIndex;
/**
* / Index of the application instance.
*/
@ProtoField(tag = 11, type = STRING)
public final String instanceId;
public HttpStart(Long timestamp, UUID requestId, PeerType peerType, Method method, String uri, String remoteAddress, String userAgent, UUID parentRequestId, UUID applicationId, Integer instanceIndex, String instanceId) {
this.timestamp = timestamp;
this.requestId = requestId;
this.peerType = peerType;
this.method = method;
this.uri = uri;
this.remoteAddress = remoteAddress;
this.userAgent = userAgent;
this.parentRequestId = parentRequestId;
this.applicationId = applicationId;
this.instanceIndex = instanceIndex;
this.instanceId = instanceId;
}
private HttpStart(Builder builder) {
this(builder.timestamp, builder.requestId, builder.peerType, builder.method, builder.uri, builder.remoteAddress, builder.userAgent, builder.parentRequestId, builder.applicationId, builder.instanceIndex, builder.instanceId);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof HttpStart)) return false;
HttpStart o = (HttpStart) other;
return equals(timestamp, o.timestamp)
&& equals(requestId, o.requestId)
&& equals(peerType, o.peerType)
&& equals(method, o.method)
&& equals(uri, o.uri)
&& equals(remoteAddress, o.remoteAddress)
&& equals(userAgent, o.userAgent)
&& equals(parentRequestId, o.parentRequestId)
&& equals(applicationId, o.applicationId)
&& equals(instanceIndex, o.instanceIndex)
&& equals(instanceId, o.instanceId);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = timestamp != null ? timestamp.hashCode() : 0;
result = result * 37 + (requestId != null ? requestId.hashCode() : 0);
result = result * 37 + (peerType != null ? peerType.hashCode() : 0);
result = result * 37 + (method != null ? method.hashCode() : 0);
result = result * 37 + (uri != null ? uri.hashCode() : 0);
result = result * 37 + (remoteAddress != null ? remoteAddress.hashCode() : 0);
result = result * 37 + (userAgent != null ? userAgent.hashCode() : 0);
result = result * 37 + (parentRequestId != null ? parentRequestId.hashCode() : 0);
result = result * 37 + (applicationId != null ? applicationId.hashCode() : 0);
result = result * 37 + (instanceIndex != null ? instanceIndex.hashCode() : 0);
result = result * 37 + (instanceId != null ? instanceId.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public Long timestamp;
public UUID requestId;
public PeerType peerType;
public Method method;
public String uri;
public String remoteAddress;
public String userAgent;
public UUID parentRequestId;
public UUID applicationId;
public Integer instanceIndex;
public String instanceId;
public Builder() {
}
public Builder(HttpStart message) {
super(message);
if (message == null) return;
this.timestamp = message.timestamp;
this.requestId = message.requestId;
this.peerType = message.peerType;
this.method = message.method;
this.uri = message.uri;
this.remoteAddress = message.remoteAddress;
this.userAgent = message.userAgent;
this.parentRequestId = message.parentRequestId;
this.applicationId = message.applicationId;
this.instanceIndex = message.instanceIndex;
this.instanceId = message.instanceId;
}
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / UNIX timestamp (in nanoseconds) when the request was sent (by a client) or received (by a server).
*/
public Builder requestId(UUID requestId) {
this.requestId = requestId;
return this;
}
/**
* / ID for tracking lifecycle of request.
*/
public Builder peerType(PeerType peerType) {
this.peerType = peerType;
return this;
}
/**
* / Role of the emitting process in the request cycle.
*/
public Builder method(Method method) {
this.method = method;
return this;
}
/**
* / Method of the request.
*/
public Builder uri(String uri) {
this.uri = uri;
return this;
}
/**
* / Destination of the request.
*/
public Builder remoteAddress(String remoteAddress) {
this.remoteAddress = remoteAddress;
return this;
}
/**
* / Remote address of the request. (For a server, this should be the origin of the request.)
*/
public Builder userAgent(String userAgent) {
this.userAgent = userAgent;
return this;
}
/**
* / Contents of the UserAgent header on the request.
*/
public Builder parentRequestId(UUID parentRequestId) {
this.parentRequestId = parentRequestId;
return this;
}
/**
* / If this request was made in order to service an incoming request, this field should track the ID of the parent.
*/
public Builder applicationId(UUID applicationId) {
this.applicationId = applicationId;
return this;
}
/**
* / If this request was made in relation to an appliciation, this field should track that application's ID.
*/
public Builder instanceIndex(Integer instanceIndex) {
this.instanceIndex = instanceIndex;
return this;
}
/**
* / Index of the application instance.
*/
public Builder instanceId(String instanceId) {
this.instanceId = instanceId;
return this;
}
@Override
public HttpStart build() {
checkRequiredFields();
return new HttpStart(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy