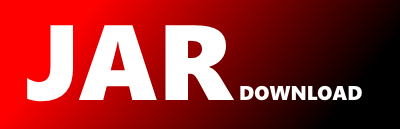
events.LogMessage Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/log.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoEnum;
import com.squareup.wire.ProtoField;
import okio.ByteString;
import static com.squareup.wire.Message.Datatype.BYTES;
import static com.squareup.wire.Message.Datatype.ENUM;
import static com.squareup.wire.Message.Datatype.INT64;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / A LogMessage contains a "log line" and associated metadata.
*/
public final class LogMessage extends Message {
public static final ByteString DEFAULT_MESSAGE = ByteString.EMPTY;
public static final MessageType DEFAULT_MESSAGE_TYPE = MessageType.OUT;
public static final Long DEFAULT_TIMESTAMP = 0L;
public static final String DEFAULT_APP_ID = "";
public static final String DEFAULT_SOURCE_TYPE = "";
public static final String DEFAULT_SOURCE_INSTANCE = "";
@ProtoField(tag = 1, type = BYTES, label = REQUIRED)
public final ByteString message;
/**
* / Bytes of the log message. (Note that it is not required to be a single line.)
*/
@ProtoField(tag = 2, type = ENUM, label = REQUIRED)
public final MessageType message_type;
/**
* / Type of the message (OUT or ERR).
*/
@ProtoField(tag = 3, type = INT64, label = REQUIRED)
public final Long timestamp;
/**
* / UNIX timestamp (in nanoseconds) when the log was written.
*/
@ProtoField(tag = 4, type = STRING)
public final String app_id;
/**
* / Application that emitted the message (or to which the application is related).
*/
@ProtoField(tag = 5, type = STRING)
public final String source_type;
/**
* / Source of the message. For Cloud Foundry, this can be "APP", "RTR", "DEA", "STG", etc.
*/
@ProtoField(tag = 6, type = STRING)
public final String source_instance;
public LogMessage(ByteString message, MessageType message_type, Long timestamp, String app_id, String source_type, String source_instance) {
this.message = message;
this.message_type = message_type;
this.timestamp = timestamp;
this.app_id = app_id;
this.source_type = source_type;
this.source_instance = source_instance;
}
private LogMessage(Builder builder) {
this(builder.message, builder.message_type, builder.timestamp, builder.app_id, builder.source_type, builder.source_instance);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof LogMessage)) return false;
LogMessage o = (LogMessage) other;
return equals(message, o.message)
&& equals(message_type, o.message_type)
&& equals(timestamp, o.timestamp)
&& equals(app_id, o.app_id)
&& equals(source_type, o.source_type)
&& equals(source_instance, o.source_instance);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = message != null ? message.hashCode() : 0;
result = result * 37 + (message_type != null ? message_type.hashCode() : 0);
result = result * 37 + (timestamp != null ? timestamp.hashCode() : 0);
result = result * 37 + (app_id != null ? app_id.hashCode() : 0);
result = result * 37 + (source_type != null ? source_type.hashCode() : 0);
result = result * 37 + (source_instance != null ? source_instance.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public ByteString message;
public MessageType message_type;
public Long timestamp;
public String app_id;
public String source_type;
public String source_instance;
public Builder() {
}
public Builder(LogMessage message) {
super(message);
if (message == null) return;
this.message = message.message;
this.message_type = message.message_type;
this.timestamp = message.timestamp;
this.app_id = message.app_id;
this.source_type = message.source_type;
this.source_instance = message.source_instance;
}
public Builder message(ByteString message) {
this.message = message;
return this;
}
/**
* / Bytes of the log message. (Note that it is not required to be a single line.)
*/
public Builder message_type(MessageType message_type) {
this.message_type = message_type;
return this;
}
/**
* / Type of the message (OUT or ERR).
*/
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / UNIX timestamp (in nanoseconds) when the log was written.
*/
public Builder app_id(String app_id) {
this.app_id = app_id;
return this;
}
/**
* / Application that emitted the message (or to which the application is related).
*/
public Builder source_type(String source_type) {
this.source_type = source_type;
return this;
}
/**
* / Source of the message. For Cloud Foundry, this can be "APP", "RTR", "DEA", "STG", etc.
*/
public Builder source_instance(String source_instance) {
this.source_instance = source_instance;
return this;
}
@Override
public LogMessage build() {
checkRequiredFields();
return new LogMessage(this);
}
}
public enum MessageType
implements ProtoEnum {
OUT(1),
ERR(2);
private final int value;
private MessageType(int value) {
this.value = value;
}
@Override
public int getValue() {
return value;
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy