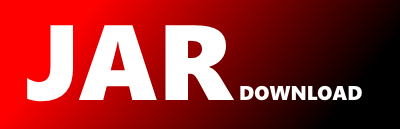
events.ValueMetric Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /Users/mheath/projects/snotel/target/checkout/client/src/main/proto/events/metric.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.DOUBLE;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / A ValueMetric indicates the value of a metric at an instant in time.
*/
public final class ValueMetric extends Message {
public static final String DEFAULT_NAME = "";
public static final Double DEFAULT_VALUE = 0D;
public static final String DEFAULT_UNIT = "";
@ProtoField(tag = 1, type = STRING, label = REQUIRED)
public final String name;
/**
* / Name of the metric. Must be consistent for downstream consumers to associate events semantically.
*/
@ProtoField(tag = 2, type = DOUBLE, label = REQUIRED)
public final Double value;
/**
* / Value at the time of event emission.
*/
@ProtoField(tag = 3, type = STRING, label = REQUIRED)
public final String unit;
public ValueMetric(String name, Double value, String unit) {
this.name = name;
this.value = value;
this.unit = unit;
}
private ValueMetric(Builder builder) {
this(builder.name, builder.value, builder.unit);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof ValueMetric)) return false;
ValueMetric o = (ValueMetric) other;
return equals(name, o.name)
&& equals(value, o.value)
&& equals(unit, o.unit);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = name != null ? name.hashCode() : 0;
result = result * 37 + (value != null ? value.hashCode() : 0);
result = result * 37 + (unit != null ? unit.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public String name;
public Double value;
public String unit;
public Builder() {
}
public Builder(ValueMetric message) {
super(message);
if (message == null) return;
this.name = message.name;
this.value = message.value;
this.unit = message.unit;
}
public Builder name(String name) {
this.name = name;
return this;
}
/**
* / Name of the metric. Must be consistent for downstream consumers to associate events semantically.
*/
public Builder value(Double value) {
this.value = value;
return this;
}
/**
* / Value at the time of event emission.
*/
public Builder unit(String unit) {
this.unit = unit;
return this;
}
@Override
public ValueMetric build() {
checkRequiredFields();
return new ValueMetric(this);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy