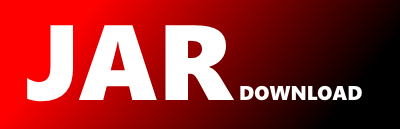
events.HttpStop Maven / Gradle / Ivy
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: /private/tmp/snotel/target/checkout/client/src/main/proto/events/http.proto
package events;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoField;
import static com.squareup.wire.Message.Datatype.ENUM;
import static com.squareup.wire.Message.Datatype.INT32;
import static com.squareup.wire.Message.Datatype.INT64;
import static com.squareup.wire.Message.Datatype.STRING;
import static com.squareup.wire.Message.Label.REQUIRED;
/**
* / An HttpStop event is emitted when a client receives a response to its request (or when a server completes its handling and returns a response).
*/
public final class HttpStop extends Message {
public static final Long DEFAULT_TIMESTAMP = 0L;
public static final String DEFAULT_URI = "";
public static final PeerType DEFAULT_PEERTYPE = PeerType.Client;
public static final Integer DEFAULT_STATUSCODE = 0;
public static final Long DEFAULT_CONTENTLENGTH = 0L;
@ProtoField(tag = 1, type = INT64, label = REQUIRED)
public final Long timestamp;
/**
* / UNIX timestamp (in nanoseconds) when the request was received.
*/
@ProtoField(tag = 2, type = STRING, label = REQUIRED)
public final String uri;
/**
* / URI of request.
*/
@ProtoField(tag = 3, label = REQUIRED)
public final UUID requestId;
/**
* / ID for tracking lifecycle of request. Should match requestId of a HttpStart event.
*/
@ProtoField(tag = 4, type = ENUM, label = REQUIRED)
public final PeerType peerType;
/**
* / Role of the emitting process in the request cycle.
*/
@ProtoField(tag = 5, type = INT32, label = REQUIRED)
public final Integer statusCode;
/**
* / Status code returned with the response to the request.
*/
@ProtoField(tag = 6, type = INT64, label = REQUIRED)
public final Long contentLength;
/**
* / Length of response (bytes).
*/
@ProtoField(tag = 7)
public final UUID applicationId;
public HttpStop(Long timestamp, String uri, UUID requestId, PeerType peerType, Integer statusCode, Long contentLength, UUID applicationId) {
this.timestamp = timestamp;
this.uri = uri;
this.requestId = requestId;
this.peerType = peerType;
this.statusCode = statusCode;
this.contentLength = contentLength;
this.applicationId = applicationId;
}
private HttpStop(Builder builder) {
this(builder.timestamp, builder.uri, builder.requestId, builder.peerType, builder.statusCode, builder.contentLength, builder.applicationId);
setBuilder(builder);
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof HttpStop)) return false;
HttpStop o = (HttpStop) other;
return equals(timestamp, o.timestamp)
&& equals(uri, o.uri)
&& equals(requestId, o.requestId)
&& equals(peerType, o.peerType)
&& equals(statusCode, o.statusCode)
&& equals(contentLength, o.contentLength)
&& equals(applicationId, o.applicationId);
}
@Override
public int hashCode() {
int result = hashCode;
if (result == 0) {
result = timestamp != null ? timestamp.hashCode() : 0;
result = result * 37 + (uri != null ? uri.hashCode() : 0);
result = result * 37 + (requestId != null ? requestId.hashCode() : 0);
result = result * 37 + (peerType != null ? peerType.hashCode() : 0);
result = result * 37 + (statusCode != null ? statusCode.hashCode() : 0);
result = result * 37 + (contentLength != null ? contentLength.hashCode() : 0);
result = result * 37 + (applicationId != null ? applicationId.hashCode() : 0);
hashCode = result;
}
return result;
}
public static final class Builder extends Message.Builder {
public Long timestamp;
public String uri;
public UUID requestId;
public PeerType peerType;
public Integer statusCode;
public Long contentLength;
public UUID applicationId;
public Builder() {
}
public Builder(HttpStop message) {
super(message);
if (message == null) return;
this.timestamp = message.timestamp;
this.uri = message.uri;
this.requestId = message.requestId;
this.peerType = message.peerType;
this.statusCode = message.statusCode;
this.contentLength = message.contentLength;
this.applicationId = message.applicationId;
}
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / UNIX timestamp (in nanoseconds) when the request was received.
*/
public Builder uri(String uri) {
this.uri = uri;
return this;
}
/**
* / URI of request.
*/
public Builder requestId(UUID requestId) {
this.requestId = requestId;
return this;
}
/**
* / ID for tracking lifecycle of request. Should match requestId of a HttpStart event.
*/
public Builder peerType(PeerType peerType) {
this.peerType = peerType;
return this;
}
/**
* / Role of the emitting process in the request cycle.
*/
public Builder statusCode(Integer statusCode) {
this.statusCode = statusCode;
return this;
}
/**
* / Status code returned with the response to the request.
*/
public Builder contentLength(Long contentLength) {
this.contentLength = contentLength;
return this;
}
/**
* / Length of response (bytes).
*/
public Builder applicationId(UUID applicationId) {
this.applicationId = applicationId;
return this;
}
@Override
public HttpStop build() {
checkRequiredFields();
return new HttpStop(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy