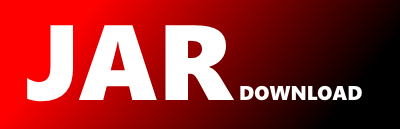
org.cloudfoundry.dropsonde.events.HttpStop Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of snotel Show documentation
Show all versions of snotel Show documentation
Java support for Cloud Foundry's Loggregator using the Dropsonde protocol.
The newest version!
// Code generated by Wire protocol buffer compiler, do not edit.
// Source file: http.proto at 80:1
package org.cloudfoundry.dropsonde.events;
import com.squareup.wire.FieldEncoding;
import com.squareup.wire.Message;
import com.squareup.wire.ProtoAdapter;
import com.squareup.wire.ProtoReader;
import com.squareup.wire.ProtoWriter;
import com.squareup.wire.WireField;
import com.squareup.wire.internal.Internal;
import java.io.IOException;
import java.lang.Integer;
import java.lang.Long;
import java.lang.Object;
import java.lang.Override;
import java.lang.String;
import java.lang.StringBuilder;
import okio.ByteString;
/**
* / An HttpStop event is emitted when a client receives a response to its request (or when a server completes its handling and returns a response).
*/
public final class HttpStop extends Message {
public static final ProtoAdapter ADAPTER = new ProtoAdapter_HttpStop();
private static final long serialVersionUID = 0L;
public static final Long DEFAULT_TIMESTAMP = 0L;
public static final String DEFAULT_URI = "";
public static final PeerType DEFAULT_PEERTYPE = PeerType.Client;
public static final Integer DEFAULT_STATUSCODE = 0;
public static final Long DEFAULT_CONTENTLENGTH = 0L;
/**
* / UNIX timestamp (in nanoseconds) when the request was received.
*/
@WireField(
tag = 1,
adapter = "com.squareup.wire.ProtoAdapter#INT64",
label = WireField.Label.REQUIRED
)
public final Long timestamp;
/**
* / URI of request.
*/
@WireField(
tag = 2,
adapter = "com.squareup.wire.ProtoAdapter#STRING",
label = WireField.Label.REQUIRED
)
public final String uri;
/**
* / ID for tracking lifecycle of request. Should match requestId of a HttpStart event.
*/
@WireField(
tag = 3,
adapter = "org.cloudfoundry.dropsonde.events.UUID#ADAPTER",
label = WireField.Label.REQUIRED
)
public final UUID requestId;
/**
* / Role of the emitting process in the request cycle.
*/
@WireField(
tag = 4,
adapter = "org.cloudfoundry.dropsonde.events.PeerType#ADAPTER",
label = WireField.Label.REQUIRED
)
public final PeerType peerType;
/**
* / Status code returned with the response to the request.
*/
@WireField(
tag = 5,
adapter = "com.squareup.wire.ProtoAdapter#INT32",
label = WireField.Label.REQUIRED
)
public final Integer statusCode;
/**
* / Length of response (bytes).
*/
@WireField(
tag = 6,
adapter = "com.squareup.wire.ProtoAdapter#INT64",
label = WireField.Label.REQUIRED
)
public final Long contentLength;
/**
* / If this request was made in relation to an appliciation, this field should track that application's ID.
*/
@WireField(
tag = 7,
adapter = "org.cloudfoundry.dropsonde.events.UUID#ADAPTER"
)
public final UUID applicationId;
public HttpStop(Long timestamp, String uri, UUID requestId, PeerType peerType, Integer statusCode, Long contentLength, UUID applicationId) {
this(timestamp, uri, requestId, peerType, statusCode, contentLength, applicationId, ByteString.EMPTY);
}
public HttpStop(Long timestamp, String uri, UUID requestId, PeerType peerType, Integer statusCode, Long contentLength, UUID applicationId, ByteString unknownFields) {
super(ADAPTER, unknownFields);
this.timestamp = timestamp;
this.uri = uri;
this.requestId = requestId;
this.peerType = peerType;
this.statusCode = statusCode;
this.contentLength = contentLength;
this.applicationId = applicationId;
}
@Override
public Builder newBuilder() {
Builder builder = new Builder();
builder.timestamp = timestamp;
builder.uri = uri;
builder.requestId = requestId;
builder.peerType = peerType;
builder.statusCode = statusCode;
builder.contentLength = contentLength;
builder.applicationId = applicationId;
builder.addUnknownFields(unknownFields());
return builder;
}
@Override
public boolean equals(Object other) {
if (other == this) return true;
if (!(other instanceof HttpStop)) return false;
HttpStop o = (HttpStop) other;
return unknownFields().equals(o.unknownFields())
&& timestamp.equals(o.timestamp)
&& uri.equals(o.uri)
&& requestId.equals(o.requestId)
&& peerType.equals(o.peerType)
&& statusCode.equals(o.statusCode)
&& contentLength.equals(o.contentLength)
&& Internal.equals(applicationId, o.applicationId);
}
@Override
public int hashCode() {
int result = super.hashCode;
if (result == 0) {
result = unknownFields().hashCode();
result = result * 37 + timestamp.hashCode();
result = result * 37 + uri.hashCode();
result = result * 37 + requestId.hashCode();
result = result * 37 + peerType.hashCode();
result = result * 37 + statusCode.hashCode();
result = result * 37 + contentLength.hashCode();
result = result * 37 + (applicationId != null ? applicationId.hashCode() : 0);
super.hashCode = result;
}
return result;
}
@Override
public String toString() {
StringBuilder builder = new StringBuilder();
builder.append(", timestamp=").append(timestamp);
builder.append(", uri=").append(uri);
builder.append(", requestId=").append(requestId);
builder.append(", peerType=").append(peerType);
builder.append(", statusCode=").append(statusCode);
builder.append(", contentLength=").append(contentLength);
if (applicationId != null) builder.append(", applicationId=").append(applicationId);
return builder.replace(0, 2, "HttpStop{").append('}').toString();
}
public static final class Builder extends Message.Builder {
public Long timestamp;
public String uri;
public UUID requestId;
public PeerType peerType;
public Integer statusCode;
public Long contentLength;
public UUID applicationId;
public Builder() {
}
/**
* / UNIX timestamp (in nanoseconds) when the request was received.
*/
public Builder timestamp(Long timestamp) {
this.timestamp = timestamp;
return this;
}
/**
* / URI of request.
*/
public Builder uri(String uri) {
this.uri = uri;
return this;
}
/**
* / ID for tracking lifecycle of request. Should match requestId of a HttpStart event.
*/
public Builder requestId(UUID requestId) {
this.requestId = requestId;
return this;
}
/**
* / Role of the emitting process in the request cycle.
*/
public Builder peerType(PeerType peerType) {
this.peerType = peerType;
return this;
}
/**
* / Status code returned with the response to the request.
*/
public Builder statusCode(Integer statusCode) {
this.statusCode = statusCode;
return this;
}
/**
* / Length of response (bytes).
*/
public Builder contentLength(Long contentLength) {
this.contentLength = contentLength;
return this;
}
/**
* / If this request was made in relation to an appliciation, this field should track that application's ID.
*/
public Builder applicationId(UUID applicationId) {
this.applicationId = applicationId;
return this;
}
@Override
public HttpStop build() {
if (timestamp == null
|| uri == null
|| requestId == null
|| peerType == null
|| statusCode == null
|| contentLength == null) {
throw Internal.missingRequiredFields(timestamp, "timestamp",
uri, "uri",
requestId, "requestId",
peerType, "peerType",
statusCode, "statusCode",
contentLength, "contentLength");
}
return new HttpStop(timestamp, uri, requestId, peerType, statusCode, contentLength, applicationId, super.buildUnknownFields());
}
}
private static final class ProtoAdapter_HttpStop extends ProtoAdapter {
ProtoAdapter_HttpStop() {
super(FieldEncoding.LENGTH_DELIMITED, HttpStop.class);
}
@Override
public int encodedSize(HttpStop value) {
return ProtoAdapter.INT64.encodedSizeWithTag(1, value.timestamp)
+ ProtoAdapter.STRING.encodedSizeWithTag(2, value.uri)
+ UUID.ADAPTER.encodedSizeWithTag(3, value.requestId)
+ PeerType.ADAPTER.encodedSizeWithTag(4, value.peerType)
+ ProtoAdapter.INT32.encodedSizeWithTag(5, value.statusCode)
+ ProtoAdapter.INT64.encodedSizeWithTag(6, value.contentLength)
+ (value.applicationId != null ? UUID.ADAPTER.encodedSizeWithTag(7, value.applicationId) : 0)
+ value.unknownFields().size();
}
@Override
public void encode(ProtoWriter writer, HttpStop value) throws IOException {
ProtoAdapter.INT64.encodeWithTag(writer, 1, value.timestamp);
ProtoAdapter.STRING.encodeWithTag(writer, 2, value.uri);
UUID.ADAPTER.encodeWithTag(writer, 3, value.requestId);
PeerType.ADAPTER.encodeWithTag(writer, 4, value.peerType);
ProtoAdapter.INT32.encodeWithTag(writer, 5, value.statusCode);
ProtoAdapter.INT64.encodeWithTag(writer, 6, value.contentLength);
if (value.applicationId != null) UUID.ADAPTER.encodeWithTag(writer, 7, value.applicationId);
writer.writeBytes(value.unknownFields());
}
@Override
public HttpStop decode(ProtoReader reader) throws IOException {
Builder builder = new Builder();
long token = reader.beginMessage();
for (int tag; (tag = reader.nextTag()) != -1;) {
switch (tag) {
case 1: builder.timestamp(ProtoAdapter.INT64.decode(reader)); break;
case 2: builder.uri(ProtoAdapter.STRING.decode(reader)); break;
case 3: builder.requestId(UUID.ADAPTER.decode(reader)); break;
case 4: {
try {
builder.peerType(PeerType.ADAPTER.decode(reader));
} catch (ProtoAdapter.EnumConstantNotFoundException e) {
builder.addUnknownField(tag, FieldEncoding.VARINT, (long) e.value);
}
break;
}
case 5: builder.statusCode(ProtoAdapter.INT32.decode(reader)); break;
case 6: builder.contentLength(ProtoAdapter.INT64.decode(reader)); break;
case 7: builder.applicationId(UUID.ADAPTER.decode(reader)); break;
default: {
FieldEncoding fieldEncoding = reader.peekFieldEncoding();
Object value = fieldEncoding.rawProtoAdapter().decode(reader);
builder.addUnknownField(tag, fieldEncoding, value);
}
}
}
reader.endMessage(token);
return builder.build();
}
@Override
public HttpStop redact(HttpStop value) {
Builder builder = value.newBuilder();
builder.requestId = UUID.ADAPTER.redact(builder.requestId);
if (builder.applicationId != null) builder.applicationId = UUID.ADAPTER.redact(builder.applicationId);
builder.clearUnknownFields();
return builder.build();
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy