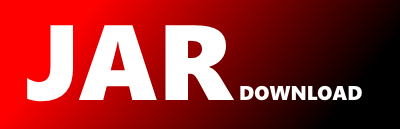
com.github.codeinghelper.jwt.JwtToken Maven / Gradle / Ivy
package com.github.codeinghelper.jwt;
import com.auth0.jwt.JWT;
import com.auth0.jwt.algorithms.Algorithm;
import com.auth0.jwt.exceptions.JWTVerificationException;
import com.auth0.jwt.interfaces.Claim;
import com.auth0.jwt.interfaces.DecodedJWT;
import com.auth0.jwt.interfaces.JWTVerifier;
import com.github.codeinghelper.util.DateUtil;
import org.springframework.beans.factory.annotation.Value;
import java.util.*;
/**
* @author :lzz
* @BelongsProject: com.github.codeinghelper.jwt
* @date :Created in 2020/8/13 11:29
* @description :
* @modified By:
*/
public class JwtToken {
public static Optional
© 2015 - 2024 Weber Informatics LLC | Privacy Policy