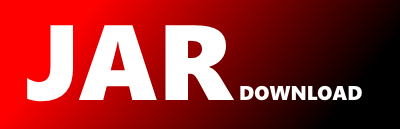
com.github.codeinghelper.exception.GlobalExceptionAdvice Maven / Gradle / Ivy
/**
* @author frank
* @version 1.0.0
* @ClassName UnifyResponse.java
* @Description 全局异常捕获
* @createTime 2020年08月01日 18:35:00
*/
package com.github.codeinghelper.exception;
import com.github.codeinghelper.configuration.ExceptionCodeConfiguration;
import com.github.codeinghelper.exception.http.ForbiddenException;
import com.github.codeinghelper.exception.http.HttpException;
import com.github.codeinghelper.exception.http.ParameterException;
import com.github.codeinghelper.exception.http.UnAuthenticatedException;
import com.github.codeinghelper.response.UnifyResponse;
import org.springframework.beans.factory.annotation.Autowired;
import org.springframework.http.HttpHeaders;
import org.springframework.http.HttpStatus;
import org.springframework.http.MediaType;
import org.springframework.http.ResponseEntity;
import org.springframework.validation.ObjectError;
import org.springframework.web.bind.MethodArgumentNotValidException;
import org.springframework.web.bind.annotation.ControllerAdvice;
import org.springframework.web.bind.annotation.ExceptionHandler;
import org.springframework.web.bind.annotation.ResponseBody;
import org.springframework.web.bind.annotation.ResponseStatus;
import javax.servlet.http.HttpServletRequest;
import javax.validation.ConstraintViolationException;
import java.util.List;
@ControllerAdvice
public class GlobalExceptionAdvice {
@Autowired
private ExceptionCodeConfiguration codeConfiguration;
@ExceptionHandler(value = Exception.class)
@ResponseBody
@ResponseStatus(code = HttpStatus.INTERNAL_SERVER_ERROR)
public UnifyResponse handleException(HttpServletRequest req, Exception e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
System.out.println(e);
UnifyResponse message = new UnifyResponse(9999, "服务器异常", method + " " + requestUrl);
return message;
}
@ExceptionHandler(HttpException.class)
public ResponseEntity handleHttpException(HttpServletRequest req, HttpException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
// ResponseEntity
UnifyResponse message = new UnifyResponse(e.getCode(), codeConfiguration.getMessage(e.getCode()), method + " " + requestUrl);
HttpHeaders headers = new HttpHeaders();
headers.setContentType(MediaType.APPLICATION_JSON_UTF8);
HttpStatus httpStatus = HttpStatus.resolve(e.getHttpStatusCode());
ResponseEntity r = new ResponseEntity<>(message, headers, httpStatus);
return r;
}
@ExceptionHandler(MethodArgumentNotValidException.class)
@ResponseBody
@ResponseStatus(code = HttpStatus.BAD_REQUEST)
public UnifyResponse handleBeanValidation(HttpServletRequest req, MethodArgumentNotValidException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
List errors = e.getBindingResult().getAllErrors();
String message = this.formatAllErrorMessages(errors);
return new UnifyResponse(10001, message, method + " " + requestUrl);
}
@ExceptionHandler(ConstraintViolationException.class)
@ResponseStatus(code = HttpStatus.BAD_REQUEST)
@ResponseBody
public UnifyResponse handleConstraintException(HttpServletRequest req, ConstraintViolationException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
String message = e.getMessage();
return new UnifyResponse(40000, message, method + " " + requestUrl);
}
@ExceptionHandler(ParameterException.class)
@ResponseStatus(code = HttpStatus.BAD_REQUEST)
@ResponseBody
public UnifyResponse handleParameterException(HttpServletRequest req, ParameterException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
String message = e.getMessage();
return new UnifyResponse(e.getCode(), codeConfiguration.getMessage(e.getCode()), method + " " + requestUrl);
}
@ExceptionHandler(UnAuthenticatedException.class)
@ResponseStatus(code = HttpStatus.UNAUTHORIZED)
@ResponseBody
public UnifyResponse handleUnAuthenticatedException(HttpServletRequest req, UnAuthenticatedException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
String message = e.getMessage();
return new UnifyResponse(e.getCode(), codeConfiguration.getMessage(e.getCode()), method + " " + requestUrl);
}
@ExceptionHandler(ForbiddenException.class)
@ResponseStatus(code = HttpStatus.FORBIDDEN)
@ResponseBody
public UnifyResponse handleForbiddenException(HttpServletRequest req, ForbiddenException e) {
String requestUrl = req.getRequestURI();
String method = req.getMethod();
return new UnifyResponse(e.getCode(), codeConfiguration.getMessage(e.getCode()), method + " " + requestUrl);
}
private String formatAllErrorMessages(List errors) {
StringBuffer errorMsg = new StringBuffer();
errors.forEach(error ->
errorMsg.append(error.getDefaultMessage()).append(';')
);
return errorMsg.toString();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy