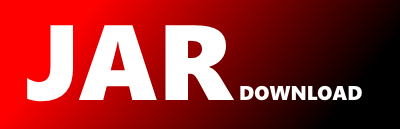
htmlcompiler.services.Http Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of htmlcompiler Show documentation
Show all versions of htmlcompiler Show documentation
An HTML compiler, with maven plugin
package htmlcompiler.services;
import com.google.gson.Gson;
import com.google.gson.reflect.TypeToken;
import com.sun.net.httpserver.HttpExchange;
import com.sun.net.httpserver.HttpHandler;
import com.sun.net.httpserver.HttpServer;
import htmlcompiler.pojos.httpmock.Endpoint;
import htmlcompiler.pojos.httpmock.Request;
import java.io.IOException;
import java.net.InetSocketAddress;
import java.nio.file.Files;
import java.nio.file.Path;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static htmlcompiler.pojos.httpmock.Endpoint.toKey;
import static htmlcompiler.pojos.httpmock.Request.toHttpHandler;
import static htmlcompiler.services.HttpHandlers.pathHandler;
import static xmlparser.utils.Functions.isNullOrEmpty;
public enum Http {;
public static HttpServer newHttpServer(final int port, final boolean requestApiEnabled
, final String requestApiSpecification, final Path... pathsToHost) throws IOException {
HttpHandler handler = pathHandler(pathsToHost);
if (requestApiEnabled) {
handler = fakeApiHandler(toApiMap(fileToSpec(requestApiSpecification), pathsToHost), handler);
}
return HttpServer
.create(new InetSocketAddress("127.0.0.1", port), 100)
.createContext("/", handler)
.getServer();
}
private static Map> fileToSpec(final String filename) throws IOException {
final String data = Files.readString(Path.of(filename));
return new Gson().fromJson(data, new TypeToken
© 2015 - 2025 Weber Informatics LLC | Privacy Policy