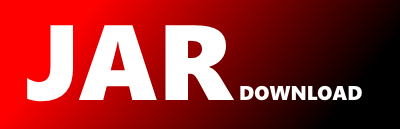
com.csoft.services.ReportBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of licensescan-maven-plugin Show documentation
Show all versions of licensescan-maven-plugin Show documentation
Maven plugin for analysing the licenses in dependencies and transitive dependencies, asserting compatibility and potentially fail the build if forbidden licenses appear
The newest version!
package com.csoft.services;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.fasterxml.jackson.databind.node.ObjectNode;
import com.github.mustachejava.DefaultMustacheFactory;
import com.github.mustachejava.Mustache;
import org.apache.maven.project.MavenProject;
import java.io.File;
import java.io.FileWriter;
import java.io.IOException;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.util.Date;
import java.util.List;
import java.util.Map;
import java.util.Set;
/**
* Class that implements generation of a LicenseScan report in the build output dir.
*/
public class ReportBuilder {
private static final String PLUGIN_NAMESPACE = "com.github.codemonstur:licensescan-maven-plugin";
private static final String REPORTING_BUILD_SUBDIR = "license-scan-results";
private static final String JSON_REPORT_FILE_NAME = "license-scan-report.json";
private static final String HTML_REPORT_TEMPLATE_NAME = "report.template.mustache";
private static final String HTML_REPORT_FILE_NAME = "index.html";
private static final ObjectMapper JSON_MAPPER = new ObjectMapper();
private static final Mustache HTML_MAPPER = new DefaultMustacheFactory().compile(HTML_REPORT_TEMPLATE_NAME);
private final MavenProject project;
public ReportBuilder(final MavenProject project) {
this.project = project;
}
/**
* Produces a Report in the build dir in JSON format.
*
* @param licenseMap artifact-to-licenses map
* @param violationsMap forbidden license -to- blocked artifact map
* @return Path of report location on filesystem.
* @throws IOException When serialization problems occur
*/
public String buildJsonReport(final Map> licenseMap,
final Map> violationsMap) throws IOException {
ObjectNode root = JSON_MAPPER.createObjectNode();
String jsonLicenseScan = JSON_MAPPER.writeValueAsString(new Results(licenseMap, violationsMap));
root.put("licenseScanResults", JSON_MAPPER.readTree(jsonLicenseScan));
Path reportPath = Files.createDirectories(
Paths.get(project.getBuild().getDirectory()).resolve(REPORTING_BUILD_SUBDIR));
File jsonReportFile = reportPath.resolve(JSON_REPORT_FILE_NAME).toFile();
JSON_MAPPER.writerWithDefaultPrettyPrinter().writeValue(jsonReportFile, root);
return jsonReportFile.toString();
}
/**
* Produces a Report in the build dir in HTML format.
*
* @param licenseMap artifact-to-licenses map
* @param violationsMap forbidden license -to- blocked artifact map
* @return Path of report location on filesystem.
* @throws IOException When serialization problems occur
*/
public String buildHtmlReport(final Map> licenseMap,
final Map> violationsMap) throws IOException {
Path reportPath = Files.createDirectories(
Paths.get(project.getBuild().getDirectory()).resolve(REPORTING_BUILD_SUBDIR));
File htmlReportFile = reportPath.resolve(HTML_REPORT_FILE_NAME).toFile();
HTML_MAPPER.execute(new FileWriter(htmlReportFile), new Report(project, licenseMap, violationsMap)).flush();
return htmlReportFile.toString();
}
/**
* Internal class used by Mustache to back the Report template.
*/
private static class Report {
private final MavenProject project;
private final Map> licenseMap;
private final Map> violationsMap;
public Report(final MavenProject project,
final Map> licenseMap,
final Map> violationsMap) {
this.project = project;
this.licenseMap = licenseMap;
this.violationsMap = violationsMap;
}
/**
* Returns the Project Name from {@link MavenProject} properties..
*
* @return String
*/
public String projectName() {
return project.getName();
}
/**
* Returns the Project Version from {@link MavenProject} properties
*
* @return String
*/
public String projectVersion() {
return project.getVersion();
}
/**
* Returns the Report execution date.
*
* @return String
*/
public String reportDate() {
return new Date().toString();
}
/**
* Returns the LicenseScan Plugin version used by the Report.
*
* @return String
*/
public String pluginVersion() {
return project.getPlugin(PLUGIN_NAMESPACE).getVersion();
}
/**
* Returns the artefacts-to-licenses entry-set.
*
* @return Set
*/
public Set>> licenses() {
return licenseMap.entrySet();
}
/**
* Returns the forbiddenLicenses-to-matchedArtefacts entry-set.
*
* @return Set
*/
public Set>> violations() {
return violationsMap.entrySet();
}
}
/**
* Internal class used to build the auxiliary Json report file.
*/
private static class Results {
public Map> licenseMap;
public Map> violationsMap;
public Results() {
}
public Results(final Map> licenseMap,
final Map> violationsMap) {
this.licenseMap = licenseMap;
this.violationsMap = violationsMap;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy