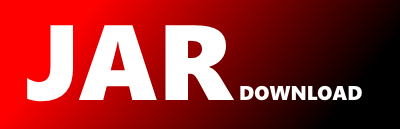
simplehttp.Handlers Maven / Gradle / Ivy
package simplehttp;
import com.sun.net.httpserver.HttpHandler;
import simplehttp.error.HttpError;
import java.security.NoSuchAlgorithmException;
import java.util.HashMap;
import java.util.Map;
import static simplehttp.ResponseBuilder.respond;
import static simplehttp.ResponseBuilder.respondError;
import static simplehttp.constants.ContentTypes.text_html;
import static simplehttp.constants.Headers.*;
import static simplehttp.constants.StatusCodes.*;
import static simplehttp.util.Characters.sha256Hex;
public enum Handlers {;
public interface HttpLogger {
void error(Exception e);
}
public static HttpHandler logError(final HttpLogger logger, final HttpHandler next) {
return exchange -> {
try {
next.handle(exchange);
} catch (Exception e) {
logger.error(e);
if (e instanceof HttpError)
((HttpError) e).processExchange(exchange);
else
respondError(exchange, INTERNAL_SERVER_ERROR, "Internal Server Error");
}
};
}
public static HttpHandler staticHtml(final String content) throws NoSuchAlgorithmException {
final String etag = String.format("\"%s\"", sha256Hex(content));
return exchange -> {
final String requestEtag = exchange.getRequestHeaders().getFirst(IF_NONE_MATCH);
if (etag.equals(requestEtag)) {
respond(exchange).status(NOT_MODIFIED).contentType(text_html).send();
} else {
respond(exchange).status(OK).contentType(text_html).header(ETAG, etag).send(content);
}
};
}
/*
public static HttpHandler resourceHandler(final String wwwroot, final HttpHandler next) {
return new ResourceHandler( new ClassPathResourceManager(Handlers.class.getClassLoader(), wwwroot), next)
.setDirectoryListingEnabled(false).setWelcomeFiles();
}
*/
public static HttpHandler html404(final String html) {
return exchange -> respond(exchange).status(NOT_FOUND).contentType(text_html).send(html);
}
public static HttpHandler redirect(final String url) {
return exchange -> respond(exchange).status(FOUND).header(LOCATION, url).send();
}
public static HttpHandler byMethod(final Map handlers) {
return byMethod(handlers, e -> respondError(e, METHOD_NOT_ALLOWED, "Method not allowed"));
}
public static HttpHandler byMethod(final Map handlers, final HttpHandler _default) {
return exchange -> handlers.getOrDefault(exchange.getRequestMethod(), _default).handle(exchange);
}
public static HttpHandler bySuffix(final Map handlers) {
return bySuffix(handlers, e -> respondError(e, NOT_FOUND, "Not Found"));
}
public static HttpHandler bySuffix(final Map handlers, final HttpHandler _default) {
return exchange -> {
final String suffix = exchange.getRequestURI().getPath().substring(exchange.getRequestURI().getPath().lastIndexOf('/'));
handlers.getOrDefault(suffix, _default).handle(exchange);
};
}
public static HttpHandler hostHeaderRouting(final Map routes, final HttpHandler fallback) {
return exchange -> {
final String host = exchange.getRequestHeaders().getFirst("Host");
final int periodIndex = (host != null) ? host.indexOf('.') : -1;
if (host == null || host.isEmpty() || periodIndex == -1) {
fallback.handle(exchange);
} else {
final String route = host.substring(0, periodIndex);
routes.getOrDefault(route, fallback).handle(exchange);
}
};
}
public static HostHeaderRoutingBuilder hostRouting() {
return new HostHeaderRoutingBuilder();
}
public static class HostHeaderRoutingBuilder {
private final Map routes = new HashMap<>();
private HttpHandler fallback = e -> respondError(e, NOT_FOUND, "Not Found");
public HostHeaderRoutingBuilder() {}
public HostHeaderRoutingBuilder prefix(final String route, final HttpHandler handler) {
this.routes.put(route, handler);
return this;
}
public HostHeaderRoutingBuilder fallback(final HttpHandler fallback) {
this.fallback = fallback;
return this;
}
public HttpHandler build() {
return hostHeaderRouting(routes, fallback);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy