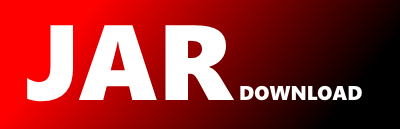
simplexml.XmlReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of simplexml Show documentation
Show all versions of simplexml Show documentation
A clean and simple XML parser, serializer, and deserializer.
package simplexml;
import simplexml.model.*;
import simplexml.utils.Interfaces.AccessDeserializers;
import java.io.IOException;
import java.io.InputStreamReader;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.util.*;
import static simplexml.utils.Constants.*;
import static simplexml.utils.Reflection.newObject;
import static simplexml.utils.Reflection.toName;
import static simplexml.utils.XML.unescapeXml;
public interface XmlReader extends AccessDeserializers {
default T domToObject(final Element node, final Class clazz) {
try {
final T o = newObject(clazz);
for (final Field f : clazz.getDeclaredFields()) {
f.setAccessible(true);
if (f.isAnnotationPresent(XmlTextNode.class)) {
final ObjectDeserializer conv = getDeserializer(f.getType());
if (conv != null) f.set(o, conv.convert(node.text));
continue;
}
final String name = toName(f);
if (f.isAnnotationPresent(XmlAttribute.class)) {
final ObjectDeserializer conv = getDeserializer(f.getType());
if (conv == null) continue;
final String value = node.attributes.get(name);
if (value == null) continue;
f.set(o, conv.convert(value));
continue;
}
final Class> type = f.getType();
if (Set.class.isAssignableFrom(type)) {
final Class> elementType = getClassOfCollection(f);
System.out.println(elementType);
final Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy