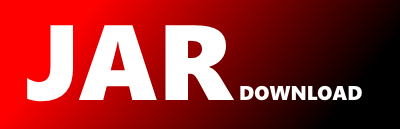
base.jee.api.cassandra.GetPersonRoles Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
A collection of basic java utility classes that provide basic features for a standalone/simple JEE application. Backed by a Cassandra, MySQL, or SQLite database, it provides, web page templates, user and group management, and a searchable online audit log of all user activity.
/**
* Creative commons Attribution-NonCommercial license.
*
* http://creativecommons.org/licenses/by-nc/2.5/au/deed.en_GB
*
* NO WARRANTY IS GIVEN OR IMPLIED, USE AT YOUR OWN RISK.
*/
package base.jee.api.cassandra;
import java.io.IOException;
import base.Query;
import base.jee.Constants;
import base.security.PermissionException;
import base.security.PersonRole;
import base.security.User;
import com.datastax.driver.core.Row;
import com.datastax.driver.core.Session;
import com.datastax.driver.core.PreparedStatement;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import static base.jee.api.cassandra.util.Log.log;
public class GetPersonRoles extends Query {
private CassandraAPI api;
private User user;
private UUID personUuid;
public GetPersonRoles(CassandraAPI api, User user, UUID personUuid) throws PermissionException {
if(api == null) {
throw new IllegalArgumentException("Invalid parameter: api");
}
if(personUuid == null) {
throw new IllegalArgumentException("Invalid parameter: personUuid");
}
if(user == null || !user.isAuthenticated()) {
throw new PermissionException(getClass().getSimpleName(), user, "Requires authenticated user.", null);
}
this.api = api;
this.user = user;
this.personUuid = personUuid;
}
public GetPersonRoles() {
}
@Override
public Query newWithParameters(Map parameters) throws PermissionException {
return new GetPersonRoles(
(CassandraAPI)parameters.get("api"),
(User)parameters.get("user"),
UUID.fromString(((String)parameters.get("personId"))));
}
public List execute() throws IOException {
List results = new LinkedList<>();
Session s = api.getCassandraSession();
if(!user.hasRole(Constants.PERSON_MANAGE_ROLE) && !personUuid.equals(user.getPersonUuid())) {
log(s, "WARN", user, "Permission denied invoking: " + GetPersonRoles.class.getSimpleName() + " " + getJsonParameters());
throw new IllegalStateException("You do not have permission to manage roles");
}
PreparedStatement p = s.prepare(
"select resource, uid, role "+
"from role " +
"where person_uuid=? allow filtering");
for(Row r : s.execute(p.bind(personUuid))) {
results.add(new PersonRole(personUuid, r.getString(0), r.getString(1), r.getString(2)));
}
return results;
}
@Override
public String getJsonParameters() {
return "{" +
"\"requesting.person\":" + user.getPersonUuid() + "," +
"\"person\":" + personUuid + "" +
"}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy