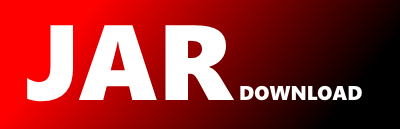
base.jee.api.sql.DeleteGroupResourceRole Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
A collection of basic java utility classes that provide basic features for a standalone/simple JEE application. Backed by a Cassandra, MySQL, or SQLite database, it provides, web page templates, user and group management, and a searchable online audit log of all user activity.
/**
* Creative commons Attribution-NonCommercial license.
*
* http://creativecommons.org/licenses/by-nc/2.5/au/deed.en_GB
*
* NO WARRANTY IS GIVEN OR IMPLIED, USE AT YOUR OWN RISK.
*/
package base.jee.api.sql;
import base.Command;
import base.jee.Constants;
import base.json.Json;
import base.security.PermissionException;
import base.security.ResourceUid;
import base.security.User;
import javax.sql.DataSource;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.UUID;
import static base.jee.api.sql.util.Log.log;
public class DeleteGroupResourceRole extends Command {
private DataSource ds;
private User user;
private UUID groupUuid;
private String resource;
private String uid;
private String role;
public DeleteGroupResourceRole(DataSource ds, User user, String resource, String uid, UUID groupUuid, String role) throws PermissionException {
if(ds == null) {
throw new IllegalArgumentException("Invalid parameter: ds");
}
if(user == null || !user.isAuthenticated()) {
throw new PermissionException(getClass().getSimpleName(), user, "Requires authenticated user.", Constants.PERSON_MANAGE_ROLE);
}
if(role == null || role.length() == 0) {
throw new IllegalArgumentException("Invalid parameter: role");
}
if(resource == null || resource.length() == 0) {
throw new IllegalArgumentException("Invalid parameter: resource");
}
if(uid == null || uid.length() == 0) {
throw new IllegalArgumentException("Invalid parameter: uid");
}
if(groupUuid == null) {
throw new IllegalArgumentException("Invalid parameter: groupUuid");
}
if(role.trim().length() > Constants.MAX_ROLE_NAME_LENGTH) {
throw new IllegalArgumentException("Role names should not have more than " + Constants.MAX_ROLE_NAME_LENGTH + " characters.");
}
if(resource.trim().length() > Constants.MAX_ROLE_RESOURCE_LENGTH) {
throw new IllegalArgumentException("Role resources should not contain more than " + Constants.MAX_ROLE_RESOURCE_LENGTH + " characters.");
}
if(uid.trim().length() > Constants.MAX_ROLE_RESOURCE_UID_LENGTH) {
throw new IllegalArgumentException("Role resources UID should not contain more than " + Constants.MAX_ROLE_RESOURCE_UID_LENGTH + " characters.");
}
this.ds = ds;
this.groupUuid = groupUuid;
this.role = role.trim();
this.resource = resource.trim();
this.uid = uid.trim();
this.user = user;
}
public DeleteGroupResourceRole() {
}
@Override
protected void execute() throws IOException {
Connection c = null;
PreparedStatement s = null;
PreparedStatement u = null;
try {
c = ds.getConnection();
c.setAutoCommit(false);
if(!user.hasRole(Constants.GROUP_MANAGE_ROLE)) {
c.rollback();
log(c, "WARN", user, "Permission denied invoking: " + DeleteGroupResourceRole.class.getSimpleName() + " " + getJsonParameters());
c.commit();
throw new PermissionException(this.getClass().getSimpleName(), user, "You do not have permission to manage roles.", Constants.GROUP_MANAGE_ROLE);
}
s = c.prepareStatement("delete from role where resource_type=? and resource_uid=? and role=? and assignee_uuid=?");
s.setString(1, resource);
s.setString(2, uid);
s.setString(3, role);
s.setString(4, groupUuid.toString());
int count = s.executeUpdate();
s.close();
s = null;
if(count > 0) {
u = c.prepareStatement("update token set roles='!' where person_uuid in (select person_uuid from group_member where group_uuid=?)");
u.setString(1, groupUuid.toString());
count = u.executeUpdate();
u.close();
u = null;
log(c, "INFO", user, "Removed role \"" + Json.escape(role) + "\" with resource \"" + Json.escape(resource) + "." + Json.escape(uid) + "\" from group \"" + Json.escape(groupUuid.toString()) + "\".", new ResourceUid("Group", groupUuid.toString()), new ResourceUid(resource, uid));
}
c.commit();
c.close();
c = null;
} catch(SQLException e) {
throw new IOException(e);
} finally {
if(s != null) { try { s.close(); } catch(SQLException e) { } }
if(u != null) { try { u.close(); } catch(SQLException e) { } }
if(c != null) {
try { c.rollback(); } catch (SQLException e) { }
try { c.close(); } catch (SQLException e) { }
}
}
}
@Override
public String getJsonParameters() {
return "{" +
"\"person\":\"" + user.getPersonUuid() + "\"," +
"\"role.group.uuid\":\"" + groupUuid + "\"," +
"\"role\":\"" + Json.escape(role) + "\"," +
"\"resource\":\"" + Json.escape(resource) + "\"," +
"\"uid\":\"" + Json.escape(uid) + "\"" +
"}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy