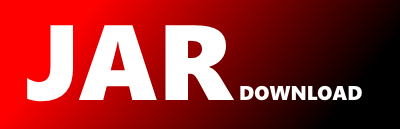
base.jee.api.sql.ExpireLogEntries Maven / Gradle / Ivy
/**
* Creative commons Attribution-NonCommercial license.
*
* http://creativecommons.org/licenses/by-nc/2.5/au/deed.en_GB
*
* NO WARRANTY IS GIVEN OR IMPLIED, USE AT YOUR OWN RISK.
*/
package base.jee.api.sql;
import base.Command;
import base.jee.api.Settings;
import javax.sql.DataSource;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import java.util.Date;
import static base.jee.api.sql.util.Log.log;
public class ExpireLogEntries extends Command {
private DataSource ds;
private Settings settings;
public ExpireLogEntries(DataSource ds, Settings settings) {
this.ds = ds;
this.settings = settings;
}
public ExpireLogEntries() {
}
@Override
protected void execute() throws IOException {
Connection c = null;
PreparedStatement s = null;
try {
c = ds.getConnection();
s = c.prepareStatement("delete from audit_event where level='DEBUG' and event_time");
s.setLong(1, new Date().getTime() - Long.parseLong(settings.get("log.max_age.debug"))*1000);
int count = s.executeUpdate();
s.close();
s = null;
c.commit();
s = c.prepareStatement("delete from audit_event where event_time");
s.setLong(1, new Date().getTime() - Long.parseLong(settings.get("log.max_age"))*1000);
count = count + s.executeUpdate();
s.close();
s = null;
c.commit();
// Remove any audit_type records that were associated with now deleted audit_event records. This is
// a potentially expensive SQL operation, might need revising in the future.
s = c.prepareStatement("delete from audit_type where audit_event_uuid in (select audit_event_uuid from audit_type left join audit_event on (audit_event_uuid=uuid) where id is null)");
s.executeUpdate();
s.close();
s = null;
if(count > 0) {
log(c, "FINE", null, "Removed " + count + " old log messages.");
}
c.commit();
c.close();
c = null;
} catch(SQLException e) {
} catch(IOException e) {
} finally {
if(s != null) { try { s.close(); } catch(SQLException e) {} }
if(c != null) { try { c.close(); } catch(SQLException e) {} }
}
}
@Override
public String getJsonParameters() {
return "{}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy