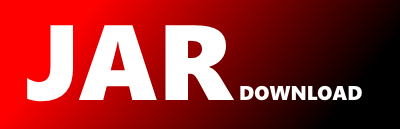
base.jee.api.sql.RecentLogEntries Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
A collection of basic java utility classes that provide basic features for a standalone/simple JEE application. Backed by a Cassandra, MySQL, or SQLite database, it provides, web page templates, user and group management, and a searchable online audit log of all user activity.
/**
* Creative commons Attribution-NonCommercial license.
*
* http://creativecommons.org/licenses/by-nc/2.5/au/deed.en_GB
*
* NO WARRANTY IS GIVEN OR IMPLIED, USE AT YOUR OWN RISK.
*/
package base.jee.api.sql;
import java.io.IOException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Date;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import base.Query;
import base.jee.Constants;
import base.jee.api.model.AuditLogEntry;
import base.security.PermissionException;
import base.security.User;
import base.text.StringHelper;
import base.text.TagsToArray;
import static base.jee.api.sql.util.Log.log;
public class RecentLogEntries extends Query {
private SqlAPI api;
private User user;
private String resourceType;
private String resourceUid;
private long limit;
private boolean debug;
public RecentLogEntries(SqlAPI api, User user, String resourceType, String resourceUid, boolean debug, long limit) throws PermissionException {
this.api = api;
this.user = user;
this.resourceType = resourceType;
this.resourceUid = resourceUid;
this.limit = limit;
this.debug = debug;
if(api == null) {
throw new IllegalArgumentException("\"api\" parameter must not be null.");
}
if(user == null || !user.isAuthenticated()) {
throw new PermissionException(this.getClass().getSimpleName(), user, "Requires an authenticated user.", "");
}
if(resourceType == null && resourceUid != null) {
throw new IllegalArgumentException("resourceType must be specified if a resourceUid is specified");
}
if(resourceType != null && resourceUid == null) {
throw new IllegalArgumentException("resourceUid must be specified if a resourceType is specified");
}
if(limit == 0) {
throw new IllegalArgumentException("Please specify a limit");
}
if(limit > 200000) {
throw new IllegalArgumentException("Limit must be less than 20,000.");
}
}
public RecentLogEntries() {
}
@Override
public Query newWithParameters(Map parameters) throws PermissionException {
return new RecentLogEntries(
(SqlAPI)parameters.get("api"),
(User)parameters.get("user"),
(String)parameters.get("resource_type"),
(String)parameters.get("resource_uid"),
parameters.get("debug") != null && ((String)parameters.get("debug")).equalsIgnoreCase("true"),
Integer.parseInt((String)parameters.get("limit")));
}
public List execute() throws IOException {
List results = new LinkedList<>();
Connection c = null;
PreparedStatement s = null;
ResultSet r = null;
try {
c = api.getDataSource().getConnection();
c.setAutoCommit(false);
if(!user.hasRole(Constants.AUDIT_ROLE)) {
log(c, "WARN", user, "Permission denied invoking: " + RecentLogEntries.class.getSimpleName() + " " + getJsonParameters());
c.commit();
throw new PermissionException(this.getClass().getSimpleName(), user, "You do not have permission to access audit information.", Constants.AUDIT_ROLE);
}
// Exclude particular IP's from appearing in the general log entry search results.
String hidden = api.getSettingsCache().get("log.hidden.ip", null);
String filter = "";
if(hidden != null) {
String[] items = TagsToArray.tagsToArray(hidden);
if(items.length > 0) {
filter = "where (ip is null or (ip != '" + StringHelper.join(items, "' and ip != '") + "')) ";
}
}
// Exclude debug messages if requested
if(!debug) {
if(filter.length() == 0) {
filter = "where e.severity!='DEBUG' ";
} else {
filter = filter + " and e.severity!='DEBUG' ";
}
}
s = c.prepareStatement(
"select e.event_time,e.severity,e.person_uuid,e.message,p.first_name,p.last_name,e.ip "+
"from base_log e left join person p on (e.person_uuid = p.uuid) "+
(resourceUid != null?" join base_log_type t on (e.uuid=t.audit_event_uuid and t.resource_type=? and t.resource_uid=?) ":" ") +
filter +
"order by e.event_time desc " +
"limit " + limit);
if(resourceUid != null) {
s.setString(1, resourceType);
s.setString(2, resourceUid);
}
r = s.executeQuery();
while(r.next()) {
results.add(new AuditLogEntry(new Date(r.getLong(1)), r.getString(2), nullableUuid(r.getString(3)), r.getString(5), r.getString(6), r.getString(4), r.getString(7)));
}
r.close();
r = null;
s.close();
s = null;
c.commit();
c.close();
c = null;
} catch(SQLException e) {
throw new IOException(e);
} finally {
if(r != null) { try { r.close(); } catch(SQLException e) {} }
if(s != null) { try { s.close(); } catch(SQLException e) {} }
if(c != null) {
try { c.rollback(); } catch (SQLException e) { }
try { c.close(); } catch (SQLException e) { }
}
}
return results;
}
@Override
public String getJsonParameters() {
return "{" +
"}";
}
private UUID nullableUuid(String uuid) {
if(uuid == null || uuid.length() == 0) {
return null;
}
return UUID.fromString(uuid);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy