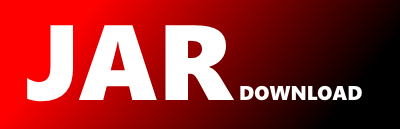
base.jee.api.sql.SignUpConfirmation Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of base Show documentation
Show all versions of base Show documentation
A collection of basic java utility classes that provide basic features for a standalone/simple JEE application. Backed by a Cassandra, MySQL, or SQLite database, it provides, web page templates, user and group management, and a searchable online audit log of all user activity.
/**
* Creative commons Attribution-NonCommercial license.
*
* http://creativecommons.org/licenses/by-nc/2.5/au/deed.en_GB
*
* NO WARRANTY IS GIVEN OR IMPLIED, USE AT YOUR OWN RISK.
*/
package base.jee.api.sql;
import java.io.IOException;
import java.security.NoSuchAlgorithmException;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.LinkedList;
import java.util.List;
import java.util.Map;
import java.util.UUID;
import base.Query;
import base.StringQueryResult;
import base.json.Json;
import base.json.JsonParser;
import base.security.PermissionException;
import base.security.ResourceUid;
import base.security.User;
import static base.jee.api.sql.util.AddPerson.addPerson;
import static base.jee.api.sql.util.CreateSession.createSession;
import static base.jee.api.sql.util.Log.log;
public class SignUpConfirmation extends Query {
private SqlAPI api;
private String requestToken;
private String ip;
public SignUpConfirmation(SqlAPI api, String requestToken, String ip) throws PermissionException {
if(api == null) {
throw new IllegalArgumentException("Invalid parameter: api");
}
if(ip == null) {
throw new IllegalArgumentException("Invalid parameter: ip");
}
if(requestToken == null || requestToken.length() == 0) {
throw new IllegalArgumentException("Must specify a request token.");
}
this.api = api;
this.ip = ip;
this.requestToken = requestToken;
}
public SignUpConfirmation() {
}
@Override
public Query newWithParameters(Map parameters) throws IOException, PermissionException {
return new SignUpConfirmation(
(SqlAPI)parameters.get("api"),
(String)parameters.get("request_token"),
(String)parameters.get("ip"));
}
@Override
protected List execute() throws IOException {
List result = new LinkedList<>();
Connection c = null;
PreparedStatement s = null;
ResultSet r = null;
try {
c = api.getDataSource().getConnection();
c.setAutoCommit(false);
s = c.prepareStatement("select data from request_token where type='signup_confirmation' and token=?");
s.setString(1, requestToken);
r = s.executeQuery();
if(!r.next()) {
throw new IllegalArgumentException("Invalid request token.");
}
JsonParser p = new JsonParser(r.getString(1));
@SuppressWarnings("unchecked")
Map data = (Map) p.parse();
r.close();
r = null;
s.close();
s = null;
String site = (String)data.get("site");
UUID uuid = addPerson(c,
site,
(String)data.get("first_name"),
(String)data.get("last_name"),
(String)data.get("email"),
(String)data.get("username"),
(String)data.get("password"),
null);
log(c, "INFO", User.userWithUuidAndIp(uuid, ip, site), "Activated self sign up account for email: " + (String)data.get("email"), new ResourceUid("Person", uuid));
int expiry = 60;
try {
expiry = Integer.parseInt(api.getSettingsCache().get("session.expiry", "3600"));
} catch(Exception e) {
}
String sessionToken = createSession(c, uuid, site, null, "", expiry);
result.add(new StringQueryResult(sessionToken));
s = c.prepareStatement("delete from request_token where type='signup_confirmation' and token=?");
s.setString(1, requestToken);
s.executeUpdate();
s.close();
s = null;
c.commit();
c.close();
c = null;
return result;
} catch(SQLException | NoSuchAlgorithmException e) {
throw new IOException(e);
} finally {
if(r != null) { try { r.close(); } catch (SQLException e) { } }
if(s != null) { try { s.close(); } catch (SQLException e) { } }
if(c != null) {
try { c.rollback(); } catch (SQLException e) { }
try { c.close(); } catch (SQLException e) { }
}
}
}
@Override
public String getJsonParameters() {
return "{" +
"\"request_token\":\"" + Json.escape(requestToken)+ "\"" +
"}";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy