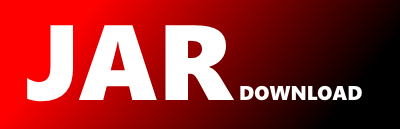
com.github.cowwoc.requirements10.java.JavaRequireThat Maven / Gradle / Ivy
/*
* Copyright (c) 2017 Gili Tzabari
* Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package com.github.cowwoc.requirements10.java;
import com.github.cowwoc.requirements10.java.validator.BigDecimalValidator;
import com.github.cowwoc.requirements10.java.validator.BigIntegerValidator;
import com.github.cowwoc.requirements10.java.validator.BooleanValidator;
import com.github.cowwoc.requirements10.java.validator.ByteValidator;
import com.github.cowwoc.requirements10.java.validator.CharacterValidator;
import com.github.cowwoc.requirements10.java.validator.CollectionValidator;
import com.github.cowwoc.requirements10.java.validator.ComparableValidator;
import com.github.cowwoc.requirements10.java.validator.DoubleValidator;
import com.github.cowwoc.requirements10.java.validator.FloatValidator;
import com.github.cowwoc.requirements10.java.validator.GenericTypeValidator;
import com.github.cowwoc.requirements10.java.validator.InetAddressValidator;
import com.github.cowwoc.requirements10.java.validator.IntegerValidator;
import com.github.cowwoc.requirements10.java.validator.ListValidator;
import com.github.cowwoc.requirements10.java.validator.LongValidator;
import com.github.cowwoc.requirements10.java.validator.MapValidator;
import com.github.cowwoc.requirements10.java.validator.ObjectArrayValidator;
import com.github.cowwoc.requirements10.java.validator.ObjectValidator;
import com.github.cowwoc.requirements10.java.validator.OptionalValidator;
import com.github.cowwoc.requirements10.java.validator.PathValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveBooleanArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveBooleanValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveByteArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveByteValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveCharacterArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveCharacterValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveDoubleArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveDoubleValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveFloatArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveFloatValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveIntegerArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveIntegerValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveLongArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveLongValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveShortArrayValidator;
import com.github.cowwoc.requirements10.java.validator.PrimitiveShortValidator;
import com.github.cowwoc.requirements10.java.validator.ShortValidator;
import com.github.cowwoc.requirements10.java.validator.StringValidator;
import com.github.cowwoc.requirements10.java.validator.UriValidator;
import java.math.BigDecimal;
import java.math.BigInteger;
import java.net.InetAddress;
import java.net.URI;
import java.nio.file.Path;
import java.util.Collection;
import java.util.List;
import java.util.Map;
import java.util.Optional;
/**
* Creates validators for the Java API that throw exceptions immediately on validation failure.
*/
public interface JavaRequireThat
{
/**
* Validates the state of a {@code byte}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveByteValidator requireThat(byte value, String name);
/**
* Validates the state of a {@code Byte}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
ByteValidator requireThat(Byte value, String name);
/**
* Validates the state of a {@code short}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveShortValidator requireThat(short value, String name);
/**
* Validates the state of a {@code Short}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
ShortValidator requireThat(Short value, String name);
/**
* Validates the state of an {@code int}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveIntegerValidator requireThat(int value, String name);
/**
* Validates the state of an {@code Integer}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
IntegerValidator requireThat(Integer value, String name);
/**
* Validates the state of a {@code long}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveLongValidator requireThat(long value, String name);
/**
* Validates the state of a {@code Long}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
LongValidator requireThat(Long value, String name);
/**
* Validates the state of a {@code float}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveFloatValidator requireThat(float value, String name);
/**
* Validates the state of a {@code Float}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
FloatValidator requireThat(Float value, String name);
/**
* Validates the state of a {@code double}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace, or is empty
*/
PrimitiveDoubleValidator requireThat(double value, String name);
/**
* Validates the state of a {@code Double}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
DoubleValidator requireThat(Double value, String name);
/**
* Validates the state of a {@code boolean}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveBooleanValidator requireThat(boolean value, String name);
/**
* Validates the state of a {@code Boolean}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
BooleanValidator requireThat(Boolean value, String name);
/**
* Validates the state of a {@code char}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveCharacterValidator requireThat(char value, String name);
/**
* Validates the state of a {@code Character}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
CharacterValidator requireThat(Character value, String name);
/**
* Validates the state of a {@code BigInteger}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
BigIntegerValidator requireThat(BigInteger value, String name);
/**
* Validates the state of a {@code BigDecimal}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
BigDecimalValidator requireThat(BigDecimal value, String name);
/**
* Validates the state of a {@code Comparable} object.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the value
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
> ComparableValidator requireThat(T value, String name);
/**
* Validates the state of an {@code Object}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the value
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
ObjectValidator requireThat(T value, String name);
/**
* Validates the state of a {@code Collection}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the value
* @param the type of elements in the collection
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
, E> CollectionValidator requireThat(T value, String name);
/**
* Validates the state of a {@code List}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the value
* @param the type of elements in the list
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
, E> ListValidator requireThat(T value, String name);
/**
* Validates the state of a primitive {@code byte} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveByteArrayValidator requireThat(byte[] value, String name);
/**
* Validates the state of a primitive {@code short} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveShortArrayValidator requireThat(short[] value, String name);
/**
* Validates the state of a primitive {@code int} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveIntegerArrayValidator requireThat(int[] value, String name);
/**
* Validates the state of a primitive {@code long} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveLongArrayValidator requireThat(long[] value, String name);
/**
* Validates the state of a primitive {@code float} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveFloatArrayValidator requireThat(float[] value, String name);
/**
* Validates the state of a primitive {@code double} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveDoubleArrayValidator requireThat(double[] value, String name);
/**
* Validates the state of a primitive {@code boolean} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveBooleanArrayValidator requireThat(boolean[] value, String name);
/**
* Validates the state of a primitive {@code char} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PrimitiveCharacterArrayValidator requireThat(char[] value, String name);
/**
* Validates the state of an {@code Object} array.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of elements in the array
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
ObjectArrayValidator requireThat(E[] value, String name);
/**
* Validates the state of a {@code Map}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of keys in the map
* @param the type of values in the map
* @param the type of the map
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
, K, V> MapValidator requireThat(T value, String name);
/**
* Validates the state of a {@code Path}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
PathValidator requireThat(Path value, String name);
/**
* Validates the state of a {@code String}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
StringValidator requireThat(String value, String name);
/**
* Validates the state of a {@code URI}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
UriValidator requireThat(URI value, String name);
/**
* Validates the state of a {@code Class}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the class modelled by the {@code Class} object. For types that contain
* type-parameters, use the {@link #requireThat(GenericType, String) TypeToken} overload.
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
GenericTypeValidator requireThat(Class value, String name);
/**
* Validates the state of a {@code Class}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of the class modelled by the {@code Class} object. For types without
* type-parameters, prefer the {@link #requireThat(Class, String) Class} overload.
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
GenericTypeValidator requireThat(GenericType value, String name);
/**
* Validates the state of an {@code Optional}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param the type of optional
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
@SuppressWarnings("OptionalUsedAsFieldOrParameterType")
OptionalValidator requireThat(Optional value, String name);
/**
* Validates the state of an {@code InetAddress}.
*
* The returned validator throws an exception immediately if a validation fails.
*
* @param value the value
* @param name the name of the value
* @return a validator for the value
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if {@code name} contains whitespace or is empty
*/
InetAddressValidator requireThat(InetAddress value, String name);
}