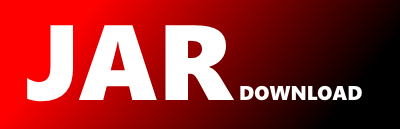
com.github.cowwoc.requirements10.java.validator.PrimitiveCharacterArrayValidator Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2019 Gili Tzabari
* Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package com.github.cowwoc.requirements10.java.validator;
import com.github.cowwoc.requirements10.java.validator.component.ArrayComponent;
import com.github.cowwoc.requirements10.java.validator.component.ObjectComponent;
import com.github.cowwoc.requirements10.java.validator.component.ValidatorComponent;
import java.util.Comparator;
/**
* Validates the state of a {@code char[]}.
*/
public interface PrimitiveCharacterArrayValidator extends
ValidatorComponent,
ObjectComponent,
ArrayComponent
{
/**
* Ensures that the array contains an element.
*
* @param expected the element
* @return this
* @throws NullPointerException if the value is null
* @throws IllegalArgumentException if the array does not contain {@code expected}
*/
PrimitiveCharacterArrayValidator contains(char expected);
/**
* Ensures that the array contains an element.
*
* @param expected the element
* @param name the name of the element
* @return this
* @throws NullPointerException if the value or {@code name} are null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the array does not contain {@code expected}
*
*/
PrimitiveCharacterArrayValidator contains(char expected, String name);
/**
* Ensures that the array does not contain {@code unwanted}.
*
* @param unwanted the unwanted element
* @return this
* @throws NullPointerException if the value is null
* @throws IllegalArgumentException if the array contains {@code unwanted}
*/
PrimitiveCharacterArrayValidator doesNotContain(char unwanted);
/**
* Ensures that the array does not contain {@code unwanted}.
*
* @param unwanted the unwanted element
* @param name the name of the unwanted element
* @return this
* @throws NullPointerException if the value or {@code name} are null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the array contains {@code unwanted}
*
*/
PrimitiveCharacterArrayValidator doesNotContain(char unwanted, String name);
/**
* Ensures that the array is sorted by its natural ordering.
*
* @return this
* @throws NullPointerException if the value is null
* @see Comparator#naturalOrder()
*/
PrimitiveCharacterArrayValidator isSorted();
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy