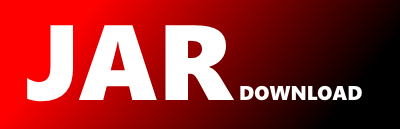
com.github.cowwoc.requirements10.java.validator.PrimitiveLongValidator Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2019 Gili Tzabari
* Licensed under the Apache License, Version 2.0: http://www.apache.org/licenses/LICENSE-2.0
*/
package com.github.cowwoc.requirements10.java.validator;
import com.github.cowwoc.requirements10.annotation.CheckReturnValue;
import com.github.cowwoc.requirements10.java.validator.component.ComparableComponent;
import com.github.cowwoc.requirements10.java.validator.component.NegativeNumberComponent;
import com.github.cowwoc.requirements10.java.validator.component.PositiveNumberComponent;
import com.github.cowwoc.requirements10.java.validator.component.ValidatorComponent;
import com.github.cowwoc.requirements10.java.validator.component.ZeroNumberComponent;
/**
* Validates the state of a {@code long}.
*/
public interface PrimitiveLongValidator extends
ValidatorComponent,
NegativeNumberComponent,
ZeroNumberComponent,
PositiveNumberComponent,
ComparableComponent
{
/**
* Returns the value that is being validated.
*
* @return the value
* @throws IllegalStateException if a previous validation failed
*/
@CheckReturnValue
long getValue();
/**
* Returns the value that is being validated.
*
* @param defaultValue the fallback value in case of a validation failure
* @return the value, or {@code defaultValue} if a previous validation failed
*/
@CheckReturnValue
long getValueOrDefault(long defaultValue);
/**
* Ensures that the value is equal to {@code expected}.
*
* @param expected the expected value
* @return this
* @throws IllegalArgumentException if the value is not equal to {@code expected}
*/
PrimitiveLongValidator isEqualTo(long expected);
/**
* Ensures that the value is equal to {@code expected}.
*
* @param expected the expected value
* @param name the name of the expected value
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is not equal to {@code expected}
*
*/
PrimitiveLongValidator isEqualTo(long expected, String name);
/**
* Ensures that the value is not equal to {@code unwanted}.
*
* @param unwanted the value to compare to
* @return this
* @throws IllegalArgumentException if the value is equal to {@code unwanted}
*/
PrimitiveLongValidator isNotEqualTo(long unwanted);
/**
* Ensures that the value is not equal to {@code unwanted}.
*
* @param unwanted the value to compare to
* @param name the name of the other value
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is equal to {@code unwanted}
*
*/
PrimitiveLongValidator isNotEqualTo(long unwanted, String name);
/**
* Ensures that the value is a multiple of {@code factor}.
*
* @param factor the number being multiplied
* @return this
* @throws IllegalArgumentException if the value is not a multiple of {@code factor}
*/
PrimitiveLongValidator isMultipleOf(long factor);
/**
* Ensures that the value is a multiple of {@code factor}.
*
* @param factor the number being multiplied
* @param name the name of the factor
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is not a multiple of {@code factor}
*
*/
PrimitiveLongValidator isMultipleOf(long factor, String name);
/**
* Ensures that the value is not a multiple of {@code factor}.
*
* @param factor the number being multiplied
* @return this
* @throws IllegalArgumentException if the value is a multiple of {@code factor}
*/
PrimitiveLongValidator isNotMultipleOf(long factor);
/**
* Ensures that the value is not a multiple of {@code factor}.
*
* @param factor the number being multiplied
* @param name the name of the factor
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - {@code value} is a multiple of {@code factor}
*
*/
PrimitiveLongValidator isNotMultipleOf(long factor, String name);
/**
* Ensures that the value is less than an upper bound.
*
* @param maximumExclusive the exclusive upper bound
* @return this
* @throws IllegalArgumentException if the value is greater than or equal to {@code maximumExclusive}
*/
PrimitiveLongValidator isLessThan(long maximumExclusive);
/**
* Ensures that the value is less than an upper bound.
*
* @param maximumExclusive the exclusive upper bound
* @param name the name of the upper bound
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is greater than or equal to {@code maximumExclusive}
*
*/
PrimitiveLongValidator isLessThan(long maximumExclusive, String name);
/**
* Ensures that the value is less than or equal to a maximum value.
*
* @param maximumInclusive the inclusive upper value
* @return this
* @throws IllegalArgumentException if the value is greater than {@code maximumInclusive}
*/
PrimitiveLongValidator isLessThanOrEqualTo(long maximumInclusive);
/**
* Ensures that the value is less than or equal to a maximum value.
*
* @param maximumInclusive the maximum value
* @param name the name of the maximum value
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is greater than {@code maximumInclusive}
*
*/
PrimitiveLongValidator isLessThanOrEqualTo(long maximumInclusive, String name);
/**
* Ensures that the value is greater than or equal to a minimum value.
*
* @param minimumInclusive the minimum value
* @return this
* @throws IllegalArgumentException if the value is less than {@code minimumInclusive}
*/
PrimitiveLongValidator isGreaterThanOrEqualTo(long minimumInclusive);
/**
* Ensures that the value is greater than or equal a minimum value.
*
* @param minimumInclusive the minimum value
* @param name the name of the minimum value
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is less than {@code minimumInclusive}
*
*/
PrimitiveLongValidator isGreaterThanOrEqualTo(long minimumInclusive, String name);
/**
* Ensures that the value is greater than a lower bound.
*
* @param minimumExclusive the exclusive lower bound
* @return this
* @throws IllegalArgumentException if the value is less than or equal to {@code minimumExclusive}
*/
PrimitiveLongValidator isGreaterThan(long minimumExclusive);
/**
* Ensures that the value is greater than a lower bound.
*
* @param minimumExclusive the exclusive lower bound
* @param name the name of the lower bound
* @return this
* @throws NullPointerException if {@code name} is null
* @throws IllegalArgumentException if:
*
* - {@code name} is empty
* - {@code name} contains whitespace
* - {@code name} is already in use by the value being validated or
* the validator context
* - the value is less or equal to {@code minimumExclusive}
*
*/
PrimitiveLongValidator isGreaterThan(long minimumExclusive, String name);
/**
* Ensures that the value is within a range.
*
* @param minimumInclusive the lower bound of the range (inclusive)
* @param maximumExclusive the upper bound of the range (exclusive)
* @return this
* @throws IllegalArgumentException if:
*
* - {@code minimumInclusive} is greater than {@code maximumExclusive}
* - the value is greater than or equal to {@code maximumExclusive}
*
*/
PrimitiveLongValidator isBetween(long minimumInclusive, long maximumExclusive);
/**
* Ensures that the value is within a range.
*
* @param minimum the lower bound of the range
* @param minimumIsInclusive {@code true} if the lower bound of the range is inclusive
* @param maximum the upper bound of the range
* @param maximumIsInclusive {@code true} if the upper bound of the range is inclusive
* @return this
* @throws IllegalArgumentException if:
*
* - {@code minimum} is greater than {@code maximum}
* - {@code minimumIsInclusive} is {@code true} and the value is less
* than {@code minimum}
* - {@code minimumIsInclusive} is {@code false} and the value is less
* than or equal to {@code minimum}
* - {@code maximumIsInclusive} is {@code true} and the value is
* greater than {@code maximum}
* - {@code maximumIsInclusive} is {@code false} and the value is
* greater than or equal to {@code maximum}
*
*/
PrimitiveLongValidator isBetween(long minimum, boolean minimumIsInclusive, long maximum,
boolean maximumIsInclusive);
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy