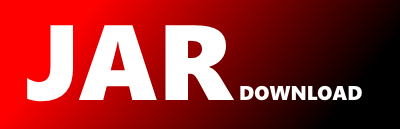
com.crabshue.commons.aws.s3.AwsS3ClientBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-aws-s3 Show documentation
Show all versions of commons-aws-s3 Show documentation
Library for Amazon AWS S3 (Simple Storage Service) integration.
The newest version!
package com.crabshue.commons.aws.s3;
import org.apache.commons.lang3.StringUtils;
import com.amazonaws.auth.AWSStaticCredentialsProvider;
import com.amazonaws.auth.BasicAWSCredentials;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.AmazonS3ClientBuilder;
import com.crabshue.commons.aws.AwsCredentials;
import com.crabshue.commons.aws.s3.domain.AwsS3Bucket;
import com.crabshue.commons.aws.s3.service.AwsS3Client;
import com.crabshue.commons.aws.s3.service.impl.AwsS3ClientImpl;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
/**
* {@link AwsS3Client} builder.
*/
@Slf4j
public class AwsS3ClientBuilder {
private AwsS3Bucket awsS3Bucket;
private AwsCredentials awsCredentials;
/**
* Prepare an {@link AwsS3Client} with a given {@link AwsS3Bucket}.
*
* @param awsS3Bucket the AWS S3 bucket
* @return new instance.
*/
public static AwsS3ClientBuilder of(@NonNull final AwsS3Bucket awsS3Bucket) {
final AwsS3ClientBuilder ret = new AwsS3ClientBuilder();
ret.awsS3Bucket = awsS3Bucket;
return ret;
}
/**
* Set credentials for {@link AwsS3Client};
*
* @param awsCredentials the credentials.
* @return instance.
*/
public AwsS3ClientBuilder withCredentials(@NonNull final AwsCredentials awsCredentials) {
this.awsCredentials = awsCredentials;
return this;
}
/**
* Build the {@link AwsS3Client}.
*
* @return the client.
*/
public AwsS3Client build() {
final AmazonS3ClientBuilder amazonS3Builder = AmazonS3ClientBuilder.standard();
if (this.awsCredentials != null && StringUtils.isNotBlank(awsCredentials.getAccessKeyId())
&& StringUtils.isNotBlank(awsCredentials.getSecretKeyId())) {
final BasicAWSCredentials awsCreds = new BasicAWSCredentials(awsCredentials.getAccessKeyId(), awsCredentials.getSecretKeyId());
amazonS3Builder.withCredentials(new AWSStaticCredentialsProvider(awsCreds));
}
amazonS3Builder.withRegion(awsS3Bucket.getRegion());
final AmazonS3 amazonS3 = amazonS3Builder.build();
logger.debug("Created AWS S3 client [{}]", amazonS3);
return new AwsS3ClientImpl(amazonS3, this.awsS3Bucket);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy