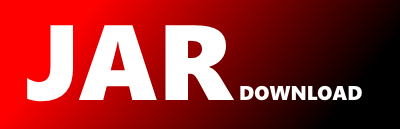
com.crabshue.commons.aws.s3.service.AwsS3Client Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of commons-aws-s3 Show documentation
Show all versions of commons-aws-s3 Show documentation
Library for Amazon AWS S3 (Simple Storage Service) integration.
The newest version!
package com.crabshue.commons.aws.s3.service;
import java.io.File;
import java.io.InputStream;
import java.util.Collection;
import com.amazonaws.services.s3.model.ObjectMetadata;
import com.amazonaws.services.s3.model.PutObjectResult;
import com.amazonaws.services.s3.model.S3ObjectSummary;
/**
* AWS S3 client.
*/
public interface AwsS3Client {
/**
* Upload a {@link File} to a certain key in the bucket.
*
* @param key the key.
* @param file the file.
* @return the result.
*/
PutObjectResult uploadObject(String key, File file);
/**
* Upload a {@link String} content to a certain key in the bucket.
*
* @param key the key.
* @param content the content.
* @return the result.
*/
PutObjectResult uploadObject(String key, String content);
/**
* Upload an {@link InputStream} content to a certain key in the bucket.
*
* @param key the key.
* @param inputStream the input stream.
* @return the result.
*/
PutObjectResult uploadObject(String key, InputStream inputStream);
/**
* Upload an {@link InputStream} content to a certain key in the bucket with given metadata.
*
* @param key the key.
* @param inputStream the input stream.
* @param objectMetadata the metadata.
* @return the result.
*/
PutObjectResult uploadObject(String key, InputStream inputStream, ObjectMetadata objectMetadata);
/**
* Delete the object located at a key in the bucket.
*
* @param key the key in the bucket.
*/
void deleteObject(String key);
/**
* Download the content located at a key in the bucket to file on the local filesystem.
*
* @param key the key.
* @param outputFile the output file.
* @return the downloaded content on the local filesystem.
*/
File downloadObject(String key, File outputFile);
/**
* List the objects in the bucket.
*
* @return the list of object descriptors.
*/
Collection listObjects();
/**
* List the objets whose key match a prefix in the bucket.
*
* @param prefix the prefix.
* @return the list of object descriptors.
*/
Collection listObjects(final String prefix);
/**
* Delete all objects located at the given keys.
*
* @param keys the keys.
*/
void deleteObjects(Collection keys);
/**
* Delete all objects in the bucket.
*/
void deleteAll();
/**
* Copy an object located at a source key to a target key.
*
* @param sourceKey the source key.
* @param targetKey the target key.
*/
void copyFile(String sourceKey, String targetKey);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy