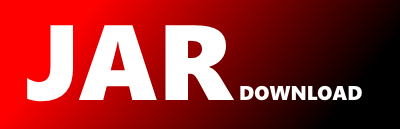
com.crabshue.commons.aws.s3.service.impl.AwsS3ClientImpl Maven / Gradle / Ivy
package com.crabshue.commons.aws.s3.service.impl;
import com.amazonaws.AmazonServiceException;
import com.amazonaws.SdkClientException;
import com.amazonaws.services.s3.AmazonS3;
import com.amazonaws.services.s3.model.*;
import com.crabshue.commons.aws.exceptions.AwsErrorType;
import com.crabshue.commons.aws.s3.domain.AwsS3Bucket;
import com.crabshue.commons.aws.s3.exceptions.AwsS3ErrorContext;
import com.crabshue.commons.aws.s3.exceptions.AwsS3ErrorType;
import com.crabshue.commons.aws.s3.service.AwsS3Client;
import com.crabshue.commons.exceptions.SystemException;
import com.crabshue.commons.file.FileIOUtils;
import edu.umd.cs.findbugs.annotations.SuppressFBWarnings;
import lombok.NonNull;
import lombok.extern.slf4j.Slf4j;
import java.io.File;
import java.io.IOException;
import java.io.InputStream;
import java.util.Collection;
import java.util.List;
import java.util.stream.Collectors;
/**
* Implementation for {@link AwsS3Client}.
*/
@Slf4j
public class AwsS3ClientImpl implements AwsS3Client {
private final AwsS3Bucket awsS3Bucket;
private final AmazonS3 amazonS3;
@SuppressFBWarnings
public AwsS3ClientImpl(final AmazonS3 amazonS3, final AwsS3Bucket awsS3Bucket) {
this.amazonS3 = amazonS3;
this.awsS3Bucket = awsS3Bucket;
}
@Override
public PutObjectResult uploadObject(@NonNull final String key,
@NonNull final File file) {
final PutObjectResult ret;
try {
ret = amazonS3.putObject(awsS3Bucket.getName(), key, file);
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_WRITE_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Uploaded file [{}] to AWS S3 bucket [{}] : [{}]", file, awsS3Bucket.getName(), ret);
return ret;
}
@Override
public PutObjectResult uploadObject(@NonNull final String key,
@NonNull final String content) {
final PutObjectResult ret;
try {
ret = amazonS3.putObject(awsS3Bucket.getName(), key, content);
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_WRITE_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Uploaded content to AWS S3 bucket [{}] : [{}]", awsS3Bucket.getName(), ret);
return ret;
}
@Override
public PutObjectResult uploadObject(@NonNull final String key,
@NonNull final InputStream inputStream) {
return uploadObject(key, inputStream, new ObjectMetadata());
}
@Override
public PutObjectResult uploadObject(@NonNull final String key,
@NonNull final InputStream inputStream,
@NonNull final ObjectMetadata objectMetadata) {
final PutObjectResult ret;
try {
ret = amazonS3.putObject(awsS3Bucket.getName(), key, inputStream, objectMetadata);
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_WRITE_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Uploaded stream to AWS S3 bucket [{}] : [{}]", awsS3Bucket.getName(), ret);
return ret;
}
@Override
public void deleteObject(@NonNull final String key) {
try {
amazonS3.deleteObject(awsS3Bucket.getName(), key);
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_DELETE_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Deleted object [{}] from AWS S3 bucket [{}]", key, awsS3Bucket.getName());
}
@Override
public File downloadObject(@NonNull final String key,
@NonNull final File outputFile) {
try {
final S3Object o = amazonS3.getObject(awsS3Bucket.getName(), key);
try (S3ObjectInputStream s3is = o.getObjectContent()) {
FileIOUtils.writeFile(s3is, outputFile);
}
} catch (AmazonServiceException | IOException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_READ_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket)
.addContextValue(AwsS3ErrorContext.KEY, key);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Downloaded object [{}] from AWS S3 bucket [{}} to [{}]", key, awsS3Bucket.getName(), outputFile);
return outputFile;
}
@Override
public Collection listObjects() {
try {
ListObjectsV2Result result = amazonS3.listObjectsV2(awsS3Bucket.getName());
final List ret = result.getObjectSummaries();
logger.info("List [{}] objects from AWS S3 bucket [{}]", ret.size(), awsS3Bucket.getName());
return ret;
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_LIST_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
}
@Override
public Collection listObjects(@NonNull final String prefix) {
try {
ListObjectsV2Result result = amazonS3.listObjectsV2(awsS3Bucket.getName(), prefix);
final List ret = result.getObjectSummaries();
logger.info("List [{}] objects with prefix [{}] from AWS S3 bucket [{}]", ret.size(), prefix, awsS3Bucket.getName());
return ret;
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_LIST_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket)
.addContextValue(AwsS3ErrorContext.PREFIX, prefix);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
}
@Override
public void deleteObjects(@NonNull final Collection keys) {
if (keys.isEmpty()) {
logger.info("AWS S3 bucket [{}] is empty. Nothing to delete.", awsS3Bucket.getName());
return;
}
final List keyVersions =
keys.stream()
.map(DeleteObjectsRequest.KeyVersion::new)
.collect(Collectors.toList());
try {
DeleteObjectsRequest dor = new DeleteObjectsRequest(awsS3Bucket.getName())
.withKeys(keyVersions);
amazonS3.deleteObjects(dor);
logger.info("Deleted [{}] objects from AWS S3 bucket [{}]", keys.size(), awsS3Bucket.getName());
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_DELETE_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket)
.addContextValue(AwsS3ErrorContext.KEY, keys);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
}
@Override
public void deleteAll() {
final List keys = this.listObjects().stream()
.map(S3ObjectSummary::getKey)
.collect(Collectors.toList());
this.deleteObjects(keys);
logger.info("Deleted all objects in AWS S3 bucket [{}]", awsS3Bucket.getName());
}
@Override
public void copyFile(@NonNull final String sourceKey,
@NonNull final String targetKey) {
try {
amazonS3.copyObject(this.awsS3Bucket.getName(), sourceKey, this.awsS3Bucket.getName(), targetKey);
} catch (AmazonServiceException e) {
throw new SystemException(AwsS3ErrorType.AWS_S3_COPY_ERROR, e)
.addContextValue(AwsS3ErrorContext.BUCKET, this.awsS3Bucket)
.addContextValue(AwsS3ErrorContext.KEY, sourceKey)
.addContextValue(AwsS3ErrorContext.KEY, targetKey);
} catch (SdkClientException e) {
throw new SystemException(AwsErrorType.AWS_SDK_CONFIGURATION_ERROR, e);
}
logger.info("Copied [{}] to [{}]", sourceKey, targetKey);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy