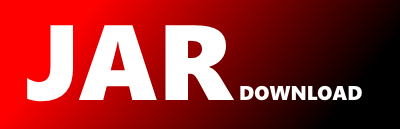
com.crabshue.commons.checksum.ChecksumCalculator.kt Maven / Gradle / Ivy
package com.crabshue.commons.checksum
import com.crabshue.commons.checksum.exceptions.ChecksumErrorContext
import com.crabshue.commons.checksum.exceptions.ChecksumErrorType
import com.crabshue.commons.exceptions.ApplicationException
import com.crabshue.commons.exceptions.SystemException
import org.apache.commons.codec.digest.DigestUtils
import java.io.File
import java.io.IOException
import java.io.InputStream
import java.nio.file.Path
import java.security.MessageDigest
import java.security.NoSuchAlgorithmException
import kotlin.io.path.exists
import kotlin.io.path.inputStream
import kotlin.io.path.pathString
class ChecksumCalculator private constructor(private val inputStream: InputStream) {
private var algorithm: String = Algorithm.MD5.type
private var toUpperCase: Boolean = false
fun withAlgorithm(algorithm: Algorithm): ChecksumCalculator {
this.algorithm = algorithm.type
return this
}
fun withAlgorithm(algorithm: String): ChecksumCalculator {
this.algorithm = algorithm
return this
}
fun withUppercase(uppercase: Boolean): ChecksumCalculator {
this.toUpperCase = uppercase
return this
}
fun computeChecksum(): String {
try {
val messageDigest = MessageDigest.getInstance(this.algorithm)
val digest = DigestUtils(messageDigest)
val hexChar = inputStream.use {
digest.digestAsHex(it)
}
return if (toUpperCase) hexChar.uppercase() else hexChar
} catch (exception: NoSuchAlgorithmException) {
throw ApplicationException(ChecksumErrorType.ALGORITHM_NOT_SUPPORTED, exception)
.addContextValue(ChecksumErrorContext.ALGORITHM, this.algorithm)
} catch (exception: IOException) {
throw SystemException(ChecksumErrorType.ERROR_READING_FILE, exception)
}
}
companion object {
@JvmStatic
fun of(byte: ByteArray): ChecksumCalculator {
val inputStream = byte.inputStream()
return ChecksumCalculator(inputStream)
}
@JvmStatic
fun of(filePath: Path): ChecksumCalculator {
if (!filePath.exists()) {
throw ApplicationException(ChecksumErrorType.FILE_NOT_EXISTS)
.addContextValue(ChecksumErrorContext.PATH, filePath.pathString)
}
return ChecksumCalculator(filePath.inputStream())
}
@JvmStatic
fun of(file: File): ChecksumCalculator {
if (!file.exists()) {
throw ApplicationException(ChecksumErrorType.FILE_NOT_EXISTS)
.addContextValue(ChecksumErrorContext.FILE, file.absolutePath)
}
return ChecksumCalculator(file.inputStream())
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy