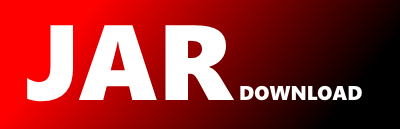
com.cryptomorin.xseries.reflection.AggregateHandle Maven / Gradle / Ivy
package com.cryptomorin.xseries.reflection;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import java.util.concurrent.Callable;
import java.util.function.Consumer;
public class AggregateHandle> implements Handle {
private final List> handles;
private Consumer handleModifier;
public AggregateHandle() {
this.handles = new ArrayList<>(5);
}
public AggregateHandle(Collection> handles) {
this.handles = new ArrayList<>(handles.size());
this.handles.addAll(handles);
}
public AggregateHandle or(H handle) {
return or(() -> handle);
}
public AggregateHandle or(Callable handle) {
this.handles.add(handle);
return this;
}
public AggregateHandle modify(Consumer handleModifier) {
this.handleModifier = handleModifier;
return this;
}
@Override
public T reflect() throws ReflectiveOperationException {
ClassNotFoundException errors = null;
for (Callable handle : handles) {
H handled;
try {
handled = handle.call();
} catch (Throwable ex) {
if (errors == null)
errors = new ClassNotFoundException("None of the aggregate handles were successful");
errors.addSuppressed(ex);
continue;
}
if (handleModifier != null) handleModifier.accept(handled);
try {
return handled.reflect();
} catch (Throwable ex) {
if (errors == null)
errors = new ClassNotFoundException("None of the aggregate handles were successful");
errors.addSuppressed(ex);
}
}
throw XReflection.relativizeSuppressedExceptions(errors);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy