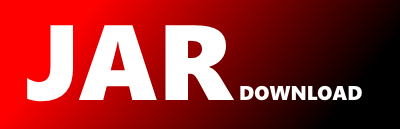
com.cryptomorin.xseries.reflection.VersionHandle Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of XSeries Show documentation
Show all versions of XSeries Show documentation
A set of utilities for Minecraft plugins
package com.cryptomorin.xseries.reflection;
import java.util.concurrent.Callable;
/**
* Provides objects based on the current version of the server ({@link XReflection#supports(int, int, int)}),
* it's mostly useful for primitive values, for reflection you should use the specialized
* {@link XReflection} class instead.
* @param the type of object to check.
*/
public final class VersionHandle {
private int version, patch;
private T handle;
// private RuntimeException errors;
VersionHandle(int version, T handle) {
this(version, 0, handle);
}
VersionHandle(int version, int patch, T handle) {
if (XReflection.supports(version, patch)) {
this.version = version;
this.patch = patch;
this.handle = handle;
}
}
public VersionHandle(int version, int patch, Callable handle) {
if (XReflection.supports(version, patch)) {
this.version = version;
this.patch = patch;
try {
this.handle = handle.call();
} catch (Exception ignored) {
}
}
}
public VersionHandle(int version, Callable handle) {
this(version, 0, handle);
}
public VersionHandle v(int version, T handle) {
return v(version, 0, handle);
}
private boolean checkVersion(int version, int patch) {
if (version == this.version && patch == this.patch)
throw new IllegalArgumentException("Cannot have duplicate version handles for version: " + version + '.' + patch);
return version > this.version && patch >= this.patch && XReflection.supports(version, patch);
}
public VersionHandle v(int version, int patch, Callable handle) {
if (!checkVersion(version, patch)) return this;
try {
this.handle = handle.call();
} catch (Exception ignored) {
}
this.version = version;
this.patch = patch;
return this;
}
public VersionHandle v(int version, int patch, T handle) {
if (checkVersion(version, patch)) {
this.version = version;
this.patch = patch;
this.handle = handle;
}
return this;
}
/**
* If none of the previous version checks matched, it'll return this object.
* @see #orElse(Callable)
*/
public T orElse(T handle) {
return this.version == 0 ? handle : this.handle;
}
/**
* If none of the previous version checks matched, it'll return this object.
* @see #orElse(Object)
*/
public T orElse(Callable handle) {
if (this.version == 0) {
try {
return handle.call();
} catch (Exception e) {
throw new RuntimeException(e);
}
}
return this.handle;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy