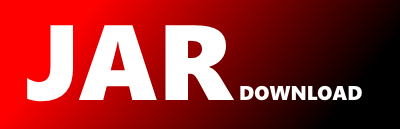
com.github.cukedoctor.parser.FeatureParser Maven / Gradle / Ivy
package com.github.cukedoctor.parser;
import com.fasterxml.jackson.core.JsonParseException;
import com.fasterxml.jackson.core.type.TypeReference;
import com.fasterxml.jackson.databind.JsonMappingException;
import com.fasterxml.jackson.databind.ObjectMapper;
import com.github.cukedoctor.api.model.Feature;
import com.github.cukedoctor.util.FileUtil;
import java.io.*;
import java.util.*;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Created by pestano on 04/06/15.
*/
public class FeatureParser {
static final Logger log = Logger.getLogger(FeatureParser.class.getName());
/**
*
* @param json absolute path to cucumber json output file
* @return list of cucumber features found in json output files
*/
public static List parse(String json) {
List features = null;
try (InputStreamReader is = new InputStreamReader(new FileInputStream(json),"UTF-8")) {
features = new ObjectMapper().readValue(is, new TypeReference>() {
});
Iterator it = features.iterator();
while (it.hasNext()) {
Feature feature = it.next();
if (!feature.isCucumberFeature()) {
log.warning("json:" + json + " is NOT a Cucumber feature result file and will be ignored");
it.remove();
}else{
feature.initScenarios();
feature.processSteps();
}
}
} catch (FileNotFoundException e) {
log.log(Level.WARNING, "Could not find json file:" + json);
} catch (JsonMappingException e) {
log.log(Level.WARNING, "Could not map json file:" + json);
} catch (JsonParseException e) {
log.log(Level.WARNING, "Could not parse json file:" + json);
} catch (IOException e) {
log.log(Level.WARNING, "Could not read json file:" + json);
}
return features;
}
/**
*
* @param paths absolute paths to cucumber json output files
* @return list of cucumber features found in json output files
*/
public static List parse(List paths) {
List features = new ArrayList<>();
for (String path : paths) {
List result = parse(path);
if (result != null) {
features.addAll(result);
}
}
return features;
}
/**
*
* @param paths absolute paths to cucumber json output files
* @return list of cucumber features found in json output files
*/
public static List parse(String... paths) {
List features = new ArrayList<>();
for (String path : paths) {
List result = parse(path);
if (result != null) {
features.addAll(result);
}
}
return features;
}
/**
*
* @param basePath relative or absolute path to a directory containing cucumber json output files
* @return list of cucumber features found in json output files
*/
public static List findAndParse(String basePath){
List paths = FileUtil.findJsonFiles(basePath);
return parse(paths);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy