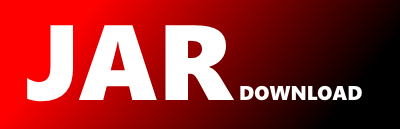
com.github.cwdtom.poseidon.socket.PoseidonSocket Maven / Gradle / Ivy
package com.github.cwdtom.poseidon.socket;
import ch.qos.logback.classic.Level;
import com.github.cwdtom.poseidon.entity.Message;
import io.netty.bootstrap.ServerBootstrap;
import io.netty.buffer.ByteBuf;
import io.netty.channel.*;
import io.netty.channel.nio.NioEventLoopGroup;
import io.netty.channel.socket.SocketChannel;
import io.netty.channel.socket.nio.NioServerSocketChannel;
import io.netty.handler.codec.ByteToMessageDecoder;
import io.netty.handler.timeout.IdleStateHandler;
import lombok.extern.slf4j.Slf4j;
import java.net.InetSocketAddress;
import java.util.List;
/**
* 监听注册端口
*
* @author chenweidong
* @since 1.0.0
*/
@Slf4j
public class PoseidonSocket implements Runnable {
private Integer port;
public PoseidonSocket(Integer port) {
this.port = port;
}
@Override
public void run() {
this.bind();
}
/**
* 绑定端口
*/
private void bind() {
EventLoopGroup boss = new NioEventLoopGroup();
EventLoopGroup worker = new NioEventLoopGroup();
ServerBootstrap bootstrap = new ServerBootstrap();
bootstrap.group(boss, worker);
bootstrap.channel(NioServerSocketChannel.class);
bootstrap.option(ChannelOption.SO_BACKLOG, 128);
bootstrap.option(ChannelOption.TCP_NODELAY, true);
bootstrap.childOption(ChannelOption.SO_KEEPALIVE, true);
bootstrap.childHandler(new ChannelInitializer() {
@Override
protected void initChannel(SocketChannel socketChannel) {
ChannelPipeline p = socketChannel.pipeline();
p.addLast(new Decode());
p.addLast(new HandlerMessage());
p.addLast(new IdleStateHandler(60, 0, 0));
p.addLast(new HeartbeatHandler());
}
});
try {
ChannelFuture channelFuture = bootstrap.bind(this.port).sync();
if (channelFuture.isSuccess()) {
log.info("poseidon is started in " + this.port);
}
channelFuture.channel().closeFuture().sync();
} catch (InterruptedException e) {
throw new RuntimeException("netty listen fail", e);
} finally {
boss.shutdownGracefully();
worker.shutdownGracefully();
}
}
/**
* 解码器
*/
private class Decode extends ByteToMessageDecoder {
@Override
protected void decode(ChannelHandlerContext channelHandlerContext, ByteBuf byteBuf, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy