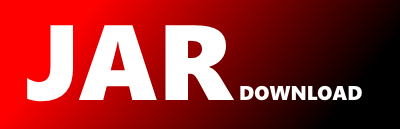
com.github.czyzby.kiwi.util.tuple.TripleTuple Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-kiwi Show documentation
Show all versions of gdx-kiwi Show documentation
Guava-inspired set of utilities for LibGDX.
package com.github.czyzby.kiwi.util.tuple;
import java.util.Map;
/** Interface shared by both mutable and immutable triple tuples. Ensures that tuples contain methods that allow to
* obtain stored values.
*
* @author MJ */
public interface TripleTuple extends Iterable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy