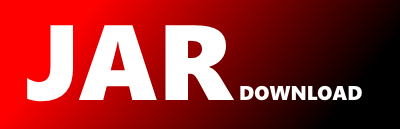
com.github.czyzby.kiwi.util.gdx.collection.GdxArrays Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-kiwi Show documentation
Show all versions of gdx-kiwi Show documentation
Guava-inspired set of utilities for LibGDX.
package com.github.czyzby.kiwi.util.gdx.collection;
import com.badlogic.gdx.utils.Array;
import com.badlogic.gdx.utils.DelayedRemovalArray;
import com.badlogic.gdx.utils.Disposable;
import com.badlogic.gdx.utils.IntArray;
import com.badlogic.gdx.utils.SnapshotArray;
import com.github.czyzby.kiwi.util.common.UtilitiesClass;
import com.github.czyzby.kiwi.util.gdx.asset.lazy.provider.ObjectProvider;
import com.github.czyzby.kiwi.util.gdx.collection.disposable.DisposableArray;
import com.github.czyzby.kiwi.util.gdx.collection.immutable.ImmutableArray;
import com.github.czyzby.kiwi.util.gdx.collection.lazy.LazyArray;
/** Common {@link Array} utilities, somewhat inspired by Guava.
*
* @author MJ */
public class GdxArrays extends UtilitiesClass {
private GdxArrays() {
}
/** @return a new, empty Array.
* @param type of stored elements. */
public static Array newArray() {
return new Array();
}
/** @param initialCapacity initial capacity of the array. Will be resized if needed.
* @return a new, empty Array.
* @param type of stored elements. */
public static Array newArray(final int initialCapacity) {
return new Array(initialCapacity);
}
/** @param forClass class of stored elements.
* @return a new, empty typed Array.
* @param type of stored elements. */
public static Array newArray(final Class forClass) {
return new Array(forClass);
}
/** @return a new, empty SnapshotArray.
* @param type of stored elements. */
public static SnapshotArray newSnapshotArray() {
return new SnapshotArray();
}
/** @param forClass class of stored elements.
* @return a new, empty typed SnapshotArray.
* @param type of stored elements. */
public static SnapshotArray newSnapshotArray(final Class forClass) {
return new SnapshotArray(forClass);
}
/** @return a new, empty DelayedRemovalArray.
* @param type of stored elements. */
public static DelayedRemovalArray newDelayedRemovalArray() {
return new DelayedRemovalArray();
}
/** @param forClass class of stored elements.
* @return a new, empty typed DelayedRemovalArray.
* @param type of stored elements. */
public static DelayedRemovalArray newDelayedRemovalArray(final Class forClass) {
return new DelayedRemovalArray(forClass);
}
/** @param values will be appended to the array.
* @return a new Array with the passed values.
* @param type of stored elements. */
public static Array newArray(final Type... values) {
return new Array(values);
}
/** @param values will be appended to the array.
* @return a new Array with the passed values.
* @param type of stored elements. */
public static Array newArray(final Iterable extends Type> values) {
final Array array = new Array();
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param forClass class of stored objects.
* @param values will be appended to the array.
* @return a new typed Array with the passed values.
* @param type of stored elements. */
public static Array newArray(final Class forClass, final Iterable extends Type> values) {
final Array array = new Array(forClass);
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param values will be appended to the array.
* @return a new SnapshotArray with the passed values.
* @param type of stored elements. */
public static SnapshotArray newSnapshotArray(final Type... values) {
return new SnapshotArray(values);
}
/** @param values will be appended to the array.
* @return a new SnapshotArray with the passed values.
* @param type of stored elements. */
public static SnapshotArray newSnapshotArray(final Iterable extends Type> values) {
final SnapshotArray array = new SnapshotArray();
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param forClass class of stored elements.
* @param values will be appended to the array.
* @return a new typed SnapshotArray with the passed values.
* @param type of stored elements. */
public static SnapshotArray newSnapshotArray(final Class forClass,
final Iterable extends Type> values) {
final SnapshotArray array = new SnapshotArray(forClass);
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param values will be appended to the array.
* @return a new DelayedRemovalArray with the passed values.
* @param type of stored elements. */
public static DelayedRemovalArray newDelayedRemovalArray(final Type... values) {
return new DelayedRemovalArray(values);
}
/** @param values will be appended to the array.
* @return a new DelayedRemovalArray with the passed values.
* @param type of stored elements. */
public static DelayedRemovalArray newDelayedRemovalArray(final Iterable extends Type> values) {
final DelayedRemovalArray array = new DelayedRemovalArray();
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param forClass class of stored elements.
* @param values will be appended to the array.
* @return a new typed DelayedRemovalArray with the passed values.
* @param type of stored elements. */
public static DelayedRemovalArray newDelayedRemovalArray(final Class forClass,
final Iterable extends Type> values) {
final DelayedRemovalArray array = new DelayedRemovalArray(forClass);
for (final Type value : values) {
array.add(value);
}
return array;
}
/** @param array will be copied.
* @return a new disposable array made using passed array values.
* @param type of stored elements. */
public static DisposableArray toDisposable(final Array extends Type> array) {
return new DisposableArray(array);
}
/** @param array will be copied.
* @return a new semi-immutable array made using passed array values.
* @param type of stored elements. */
public static ImmutableArray toImmutable(final Array extends Type> array) {
return new ImmutableArray(array);
}
/** @param array will be copied.
* @return a new snapshot array created with the passed array values.
* @param type of stored elements. */
public static SnapshotArray toSnapshot(final Array extends Type> array) {
return new SnapshotArray(array);
}
/** @param array will be copied.
* @return a new delayed removal array created with the passed array values.
* @param type of stored elements. */
public static DelayedRemovalArray toDelayedRemoval(final Array extends Type> array) {
return new DelayedRemovalArray(array);
}
/** @param array will be copied.
* @param provider creates new object on get(index) calls if the value on the selected index is null.
* @return a new lazy array created with the passed array values.
* @param type of stored elements. */
public static LazyArray toLazy(final ObjectProvider extends Type> provider,
final Array extends Type> array) {
return new LazyArray(provider, array);
}
/** @param array can be null.
* @return true if array is null or has no elements. */
public static boolean isEmpty(final Array> array) {
return array == null || array.size == 0;
}
/** @param array can be null.
* @return true if array is not null and has at least one element. */
public static boolean isNotEmpty(final Array> array) {
return array != null && array.size > 0;
}
/** @param arrays will be checked. Can be null.
* @return the biggest size among the passed arrays. 0 if all arrays are empty or null. */
public static int getBiggestSize(final Array>... arrays) {
int maxSize = 0;
for (final Array> array : arrays) {
if (array != null) {
maxSize = Math.max(maxSize, array.size);
}
}
return maxSize;
}
/** @param arrays will be checked. Can be null.
* @return the biggest size among the passed arrays. 0 if all arrays are empty or null. */
public static int getBiggestSize(final Iterable> arrays) {
int maxSize = 0;
for (final Array> array : arrays) {
if (array != null) {
maxSize = Math.max(maxSize, array.size);
}
}
return maxSize;
}
/** @param array cannot be null.
* @param index will be checked.
* @return true if the given index is last in the passed array. */
public static boolean isIndexLast(final Array> array, final int index) {
return array.size - 1 == index;
}
/** @param array cannot be null.
* @param index will be checked.
* @return true if the given index is valid for the passed array. */
public static boolean isIndexValid(final Array> array, final int index) {
return index >= 0 && index < array.size;
}
/** @param array can be null.
* @return null (if array is empty or last stored value was null) or last stored value.
* @param type of stored values. */
public static Type removeLast(final Array extends Type> array) {
if (isEmpty(array)) {
return null;
}
return array.removeIndex(array.size - 1);
}
/** @param array can be null.
* @return last stored value in the array if it is not empty or null.
* @param type of stored values. */
public static Type getLast(final Array extends Type> array) {
if (isEmpty(array)) {
return null;
}
return array.get(array.size - 1);
}
/** @param intArray can be null.
* @return last stored int in the array if it is not empty or 0. */
public static int getLast(final IntArray intArray) {
if (isEmpty(intArray)) {
return 0;
}
return intArray.get(intArray.size - 1);
}
/** @param intArray can be null.*
* @return true if the passed array is empty. */
public static boolean isEmpty(final IntArray intArray) {
return intArray == null || intArray.size == 0;
}
/** @param arrays will be joined.
* @return a new array with values from all passed arrays. Duplicates are added multiple times.
* @param type of stored elements. */
public static Array union(final Array... arrays) {
return unionTo(new Array(), arrays);
}
/** @param ofClass class of stored elements.
* @param arrays will be joined.
* @return a new typed array with values from all passed arrays. Duplicates are added multiple times.
* @param type of stored elements. */
public static Array union(final Class ofClass, final Array... arrays) {
return unionTo(new Array(ofClass), arrays);
}
/** @param array will contain passed arrays.
* @param arrays will be joined.
* @return first argument array with values added from all passed arrays. Duplicates are added multiple times.
* @param type of stored elements. */
public static Array unionTo(final Array array, final Array... arrays) {
if (arrays == null || arrays.length == 0) {
return array;
}
for (final Array unionedArray : arrays) {
array.addAll(unionedArray);
}
return array;
}
/** @param arrays will all be cleared, with an additional null-check before the clearing. */
public static void clearAll(final Array>... arrays) {
for (final Array> array : arrays) {
if (array != null) {
array.clear();
}
}
}
/** @param arrays all contained arrays will all be cleared, with an additional null-check before the clearing. */
public static void clearAll(final Iterable> arrays) {
for (final Array> array : arrays) {
if (array != null) {
array.clear();
}
}
}
/** Static utility for accessing {@link Array#size} variable (which is kind of ugly, since it allows to easily
* modify and damage internal collection data). Performs null check.
*
* @param array its size will be checked. Can be null.
* @return current size of the passed array. 0 if array is empty or null. */
public static int sizeOf(final Array> array) {
if (array == null) {
return 0;
}
return array.size;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy