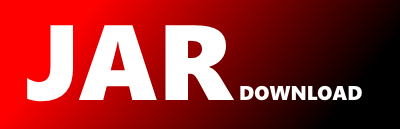
com.github.czyzby.kiwi.util.gdx.collection.GdxMaps Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-kiwi Show documentation
Show all versions of gdx-kiwi Show documentation
Guava-inspired set of utilities for LibGDX.
package com.github.czyzby.kiwi.util.gdx.collection;
import com.badlogic.gdx.utils.ArrayMap;
import com.badlogic.gdx.utils.Disposable;
import com.badlogic.gdx.utils.IdentityMap;
import com.badlogic.gdx.utils.ObjectMap;
import com.badlogic.gdx.utils.OrderedMap;
import com.github.czyzby.kiwi.util.common.UtilitiesClass;
import com.github.czyzby.kiwi.util.gdx.asset.lazy.provider.ObjectProvider;
import com.github.czyzby.kiwi.util.gdx.collection.disposable.DisposableObjectMap;
import com.github.czyzby.kiwi.util.gdx.collection.immutable.ImmutableObjectMap;
import com.github.czyzby.kiwi.util.gdx.collection.lazy.LazyObjectMap;
/** Common LibGDX maps utilities, somewhat inspired by Guava.
*
* @author MJ */
public class GdxMaps extends UtilitiesClass {
private GdxMaps() {
}
/** @return an empty, new {@link ObjectMap}.
* @param type of map keys.
* @param type of map values. */
public static ObjectMap newObjectMap() {
return new ObjectMap();
}
/** @param map will be copied.
* @return a new object map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static ObjectMap newObjectMap(final ObjectMap extends Key, ? extends Value> map) {
return new ObjectMap(map);
}
/** @param keyAndValues pairs of keys and values. Each value has to be proceeded by a key.
* @return a new object map with the given values. Not fail-fast - be careful when passing arguments, or it might
* result in unexpected map values.
* @param type of map keys.
* @param type of map values. */
@SuppressWarnings("unchecked")
public static ObjectMap newObjectMap(final Object... keyAndValues) {
if (keyAndValues.length % 2 != 0) {
throw new IllegalArgumentException("Total number of keys and values has to be even.");
}
final ObjectMap map = new ObjectMap();
for (int pairIndex = 0; pairIndex < keyAndValues.length; pairIndex++) {
map.put((Key) keyAndValues[pairIndex], (Value) keyAndValues[++pairIndex]);
}
return map;
}
/** @return an empty, new ordered map.
* @param type of map keys.
* @param type of map values. */
public static OrderedMap newOrderedMap() {
return new OrderedMap();
}
/** @param map will be copied.
* @return a new ordered map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static OrderedMap newOrderedMap(
final OrderedMap extends Key, ? extends Value> map) {
return new OrderedMap(map);
}
/** @param keyAndValues pairs of keys and values. Each value has to be proceeded by a key.
* @return a new ordered map with the given values. Not fail-fast - be careful when passing arguments, or it might
* result in unexpected map values.
* @param type of map keys.
* @param type of map values. */
@SuppressWarnings("unchecked")
public static OrderedMap newOrderedMap(final Object... keyAndValues) {
if (keyAndValues.length % 2 != 0) {
throw new IllegalArgumentException("Total number of keys and values has to be even.");
}
final OrderedMap map = new OrderedMap();
for (int pairIndex = 0; pairIndex < keyAndValues.length; pairIndex++) {
map.put((Key) keyAndValues[pairIndex], (Value) keyAndValues[++pairIndex]);
}
return map;
}
/** @return an empty, new identity map.
* @param type of map keys.
* @param type of map values. */
public static IdentityMap newIdentityMap() {
return new IdentityMap();
}
/** @param map will be copied.
* @return a new identity map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static IdentityMap newIdentityMap(
final IdentityMap extends Key, ? extends Value> map) {
return new IdentityMap(map);
}
/** @param keyAndValues pairs of keys and values. Each value has to be proceeded by a key.
* @return a new identity map with the given values. Not fail-fast - be careful when passing arguments, or it might
* result in unexpected map values.
* @param type of map keys.
* @param type of map values. */
@SuppressWarnings("unchecked")
public static IdentityMap newIdentityMap(final Object... keyAndValues) {
if (keyAndValues.length % 2 != 0) {
throw new IllegalArgumentException("Total number of keys and values has to be even.");
}
final IdentityMap map = new IdentityMap();
for (int pairIndex = 0; pairIndex < keyAndValues.length; pairIndex++) {
map.put((Key) keyAndValues[pairIndex], (Value) keyAndValues[++pairIndex]);
}
return map;
}
/** @return an empty, new array map.
* @param type of map keys.
* @param type of map values. */
public static ArrayMap newArrayMap() {
return new ArrayMap();
}
/** @param map will be copied.
* @return a new array map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static ArrayMap newArrayMap(final ArrayMap extends Key, ? extends Value> map) {
return new ArrayMap(map);
}
/** @param ordered if true, map will be ordered.
* @return an empty, new array map.
* @param type of map keys.
* @param type of map values. */
public static ArrayMap newArrayMap(final boolean ordered) {
return new ArrayMap(ordered, 16); // default capacity
}
/** @param keyType class of map keys.
* @param valueType class of map values.
* @return an empty, new typed array map.
* @param type of map keys.
* @param type of map values. */
public static ArrayMap newArrayMap(final Class keyType,
final Class valueType) {
return new ArrayMap(keyType, valueType);
}
/** @param keyAndValues pairs of keys and values. Each value has to be proceeded by a key.
* @return a new array map with the given values. Not fail-fast - be careful when passing arguments, or it might
* result in unexpected map values.
* @param type of map keys.
* @param type of map values. */
@SuppressWarnings("unchecked")
public static ArrayMap newArrayMap(final Object... keyAndValues) {
if (keyAndValues.length % 2 != 0) {
throw new IllegalArgumentException("Total number of keys and values has to be even.");
}
final ArrayMap map = new ArrayMap();
for (int pairIndex = 0; pairIndex < keyAndValues.length; pairIndex++) {
map.put((Key) keyAndValues[pairIndex], (Value) keyAndValues[++pairIndex]);
}
return map;
}
/** @param keyType class of map keys.
* @param valueType class of map values.
* @param keyAndValues pairs of keys and values. Each value has to be proceeded by a key.
* @return a new typed array map with the given values. Not fail-fast - be careful when passing arguments, or it
* might result in unexpected map values.
* @param type of map keys.
* @param type of map values. */
@SuppressWarnings("unchecked")
public static ArrayMap newArrayMap(final Class keyType, final Class valueType,
final Object... keyAndValues) {
if (keyAndValues.length % 2 != 0) {
throw new IllegalArgumentException("Total number of keys and values has to be even.");
}
final ArrayMap map = new ArrayMap(keyType, valueType);
for (int pairIndex = 0; pairIndex < keyAndValues.length; pairIndex++) {
map.put((Key) keyAndValues[pairIndex], (Value) keyAndValues[++pairIndex]);
}
return map;
}
/** @param map will be copied.
* @return a new disposable object map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static DisposableObjectMap toDisposable(
final ObjectMap extends Key, ? extends Value> map) {
return new DisposableObjectMap(map);
}
/** @param map will be copied.
* @return a new immutable map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static ImmutableObjectMap toImmutable(
final ObjectMap extends Key, ? extends Value> map) {
return new ImmutableObjectMap(map);
}
/** @param map will be copied.
* @return a new ordered map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static OrderedMap toOrdered(final ObjectMap extends Key, ? extends Value> map) {
final OrderedMap orderedMap = new OrderedMap();
for (final ObjectMap.Entry extends Key, ? extends Value> entry : map) {
orderedMap.put(entry.key, entry.value);
}
return orderedMap;
}
/** @param map will be copied.
* @param provider creates new object on get(key) calls if the key is not present in the map.
* @return a new ordered map with the passed values.
* @param type of map keys.
* @param type of map values. */
public static LazyObjectMap toLazy(final ObjectMap extends Key, ? extends Value> map,
final ObjectProvider extends Value> provider) {
return new LazyObjectMap(provider, map);
}
/** @param map can be null.
* @return true if map is null or has no elements. */
public static boolean isEmpty(final ObjectMap, ?> map) {
return map == null || map.size == 0;
}
/** @param map can be null.
* @return true if map is not null and has at least one element. */
public static boolean isNotEmpty(final ObjectMap, ?> map) {
return map != null && map.size > 0;
}
/** Puts a value with the given key in the passed map, provided that the passed key isn't already present in the
* map.
*
* @param map may contain a value associated with the key.
* @param key map key.
* @param value map value to add.
* @return value associated with the key in the map (recently added or the previous one).
* @param type of map keys.
* @param type of map values. */
public static Value putIfAbsent(final ObjectMap map, final Key key, final Value value) {
if (!map.containsKey(key)) {
map.put(key, value);
return value;
}
return map.get(key);
}
/** @param maps will all be cleared, with an additional null-check before the clearing. */
public static void clearAll(final ObjectMap, ?>... maps) {
for (final ObjectMap, ?> map : maps) {
if (map != null) {
map.clear();
}
}
}
/** @param maps all contained maps will all be cleared, with an additional null-check before the clearing. */
public static void clearAll(final Iterable> maps) {
for (final ObjectMap, ?> map : maps) {
if (map != null) {
map.clear();
}
}
}
/** Static utility for accessing {@link ObjectMap#size} variable (which is kind of ugly, since it allows to easily
* modify and damage internal collection data). Performs null check.
*
* @param map its size will be checked. Can be null.
* @return current size of the passed map. 0 if map is empty or null. */
public static int sizeOf(final ObjectMap, ?> map) {
if (map == null) {
return 0;
}
return map.size;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy