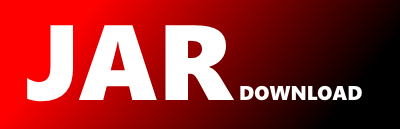
com.github.czyzby.kiwi.util.gdx.collection.disposable.DisposableObjectSet Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of gdx-kiwi Show documentation
Show all versions of gdx-kiwi Show documentation
Guava-inspired set of utilities for LibGDX.
package com.github.czyzby.kiwi.util.gdx.collection.disposable;
import com.badlogic.gdx.utils.Disposable;
import com.badlogic.gdx.utils.ObjectSet;
/** An unordered set where the keys are objects. This implementation uses cuckoo hashing using 3 hashes, random walking,
* and a small stash for problematic keys. Null keys are not allowed. No allocation is done except when growing the
* table size.
*
* This set performs very fast contains and remove (typically O(1), worst case O(log(n))). Add may be a bit slower,
* depending on hash collisions. Load factors greater than 0.91 greatly increase the chances the set will have to rehash
* to the next higher POT size.
*
* Utility container for disposable objects.
*
* @author Nathan Sweet
* @author MJ */
public class DisposableObjectSet extends ObjectSetimplements Disposable {
/** Creates a new set with an initial capacity of 32 and a load factor of 0.8. This set will hold 25 items before
* growing the backing table. */
public DisposableObjectSet() {
super();
}
/** Creates a new set with a load factor of 0.8. This set will hold initialCapacity * 0.8 items before growing the
* backing table.
*
* @param initialCapacity initial expected amount of elements. */
public DisposableObjectSet(final int initialCapacity) {
super(initialCapacity);
}
/** Creates a new set with the specified initial capacity and load factor. This set will hold initialCapacity *
* loadFactor items before growing the backing table.
*
* @param initialCapacity initial expected amount of elements.
* @param loadFactor determines how fast set is grown. */
public DisposableObjectSet(final int initialCapacity, final float loadFactor) {
super(initialCapacity, loadFactor);
}
/** Creates a new set identical to the specified set.
*
* @param set will be copied. */
public DisposableObjectSet(final ObjectSet extends Type> set) {
super(set);
}
/** @return a new instance of disposable set.
* @param type of stored values. */
public static DisposableObjectSet newSet() {
return new DisposableObjectSet();
}
/** @param disposables will be copied.
* @return a new DisposableObjectSet with the passed values.
* @param type of stored values. */
public static DisposableObjectSet of(final Type... disposables) {
final DisposableObjectSet set = new DisposableObjectSet(disposables.length);
for (final Type disposable : disposables) {
set.add(disposable);
}
return set;
}
/** @param disposables will be copied.
* @return a new DisposableObjectSet with the passed values.
* @param type of stored values. */
public static DisposableObjectSet with(final Type... disposables) {
return of(disposables);
}
/** @param objectSet will be copied.
* @return a new DisposableObjectSet with the values specified in the passed set.
* @param type of stored values. */
public static DisposableObjectSet copyOf(
final ObjectSet extends Type> objectSet) {
return new DisposableObjectSet(objectSet);
}
/** @return amount of elements in the set. */
public int size() {
return super.size;
}
@Override
public void dispose() {
for (final Disposable disposable : this) {
if (disposable != null) {
disposable.dispose();
}
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy