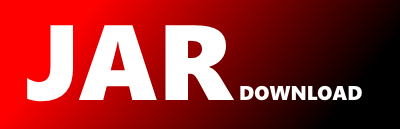
com.daxie.joglf.gl.draw.GLDrawFunctions3D Maven / Gradle / Ivy
package com.daxie.joglf.gl.draw;
import java.nio.Buffer;
import java.nio.FloatBuffer;
import java.nio.IntBuffer;
import java.util.ArrayList;
import java.util.List;
import com.daxie.basis.coloru8.ColorU8;
import com.daxie.basis.coloru8.ColorU8Functions;
import com.daxie.basis.matrix.Matrix;
import com.daxie.basis.matrix.MatrixFunctions;
import com.daxie.basis.vector.Vector;
import com.daxie.basis.vector.VectorFunctions;
import com.daxie.joglf.gl.shader.GLShaderFunctions;
import com.daxie.joglf.gl.shape.Quadrangle;
import com.daxie.joglf.gl.shape.Triangle;
import com.daxie.joglf.gl.shape.Vertex3D;
import com.daxie.joglf.gl.texture.TextureMgr;
import com.daxie.joglf.gl.wrapper.GLWrapper;
import com.daxie.log.LogFile;
import com.jogamp.common.nio.Buffers;
import com.jogamp.opengl.GL4;
/**
* Draw functions for 3D primitives
* @author Daba
*
*/
public class GLDrawFunctions3D {
public static void DrawLine3D(Vector line_pos_1,Vector line_pos_2,ColorU8 color_1,ColorU8 color_2) {
IntBuffer pos_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer color_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer vao=Buffers.newDirectIntBuffer(1);
FloatBuffer pos_buffer=Buffers.newDirectFloatBuffer(6);
FloatBuffer color_buffer=Buffers.newDirectFloatBuffer(8);
pos_buffer.put(line_pos_1.GetX());
pos_buffer.put(line_pos_1.GetY());
pos_buffer.put(line_pos_1.GetZ());
pos_buffer.put(line_pos_2.GetX());
pos_buffer.put(line_pos_2.GetY());
pos_buffer.put(line_pos_2.GetZ());
color_buffer.put(color_1.GetR());
color_buffer.put(color_1.GetG());
color_buffer.put(color_1.GetB());
color_buffer.put(color_1.GetA());
color_buffer.put(color_2.GetR());
color_buffer.put(color_2.GetG());
color_buffer.put(color_2.GetB());
color_buffer.put(color_2.GetA());
((Buffer)pos_buffer).flip();
((Buffer)color_buffer).flip();
GLShaderFunctions.UseProgram("color");
GLWrapper.glGenBuffers(1, pos_vbo);
GLWrapper.glGenBuffers(1, color_vbo);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*pos_buffer.capacity(),pos_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*color_buffer.capacity(),color_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glGenVertexArrays(1, vao);
GLWrapper.glBindVertexArray(vao.get(0));
//Position attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(0);
GLWrapper.glVertexAttribPointer(0, 3, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*3, 0);
//Color attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(1);
GLWrapper.glVertexAttribPointer(1, 4, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*4, 0);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, 0);
GLWrapper.glBindVertexArray(0);
//Draw
GLWrapper.glBindVertexArray(vao.get(0));
GLWrapper.glEnable(GL4.GL_BLEND);
GLWrapper.glDrawArrays(GL4.GL_LINES, 0, 2);
GLWrapper.glDisable(GL4.GL_BLEND);
GLWrapper.glBindVertexArray(0);
//Delete buffers
GLWrapper.glDeleteBuffers(1, pos_vbo);
GLWrapper.glDeleteBuffers(1, color_vbo);
GLWrapper.glDeleteVertexArrays(1, vao);
}
public static void DrawLine3D(Vector line_pos_1,Vector line_pos_2,ColorU8 color) {
DrawLine3D(line_pos_1, line_pos_2, color,color);
}
public static void DrawAxes(float length) {
DrawLine3D(
VectorFunctions.VGet(-length, 0.0f, 0.0f),
VectorFunctions.VGet(length, 0.0f, 0.0f),
ColorU8Functions.GetColorU8(1.0f, 0.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, -length, 0.0f),
VectorFunctions.VGet(0.0f, length, 0.0f),
ColorU8Functions.GetColorU8(0.0f, 1.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, 0.0f, -length),
VectorFunctions.VGet(0.0f, 0.0f, length),
ColorU8Functions.GetColorU8(0.0f, 0.0f, 1.0f, 1.0f));
}
public static void DrawAxes_Positive(float length) {
DrawLine3D(
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
VectorFunctions.VGet(length, 0.0f, 0.0f),
ColorU8Functions.GetColorU8(1.0f, 0.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
VectorFunctions.VGet(0.0f, length, 0.0f),
ColorU8Functions.GetColorU8(0.0f, 1.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
VectorFunctions.VGet(0.0f, 0.0f, length),
ColorU8Functions.GetColorU8(0.0f, 0.0f, 1.0f, 1.0f));
}
public static void DrawAxes_Negative(float length) {
DrawLine3D(
VectorFunctions.VGet(-length, 0.0f, 0.0f),
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
ColorU8Functions.GetColorU8(1.0f, 0.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, -length, 0.0f),
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
ColorU8Functions.GetColorU8(0.0f, 1.0f, 0.0f, 1.0f));
DrawLine3D(
VectorFunctions.VGet(0.0f, 0.0f, -length),
VectorFunctions.VGet(0.0f, 0.0f, 0.0f),
ColorU8Functions.GetColorU8(0.0f, 0.0f, 1.0f, 1.0f));
}
public static void DrawTriangle3D(Vector triangle_pos_1,Vector triangle_pos_2,Vector triangle_pos_3,ColorU8 color) {
Triangle triangle=new Triangle();
Vertex3D[] vertices=new Vertex3D[3];
for(int i=0;i<3;i++) {
vertices[i]=new Vertex3D();
}
vertices[0].SetPos(triangle_pos_1);
vertices[1].SetPos(triangle_pos_2);
vertices[2].SetPos(triangle_pos_3);
for(int i=0;i<3;i++) {
vertices[i].SetDif(color);
}
for(int i=0;i<3;i++) {
triangle.SetVertex(i, vertices[i]);
}
DrawTriangle3D(triangle);
}
public static void DrawTriangle3D(Triangle triangle) {
IntBuffer pos_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer color_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer vao=Buffers.newDirectIntBuffer(1);
Vertex3D[] vertices=triangle.GetVertices();
FloatBuffer pos_buffer=Buffers.newDirectFloatBuffer(9);
FloatBuffer color_buffer=Buffers.newDirectFloatBuffer(12);
for(int i=0;i<3;i++) {
Vector pos=vertices[i].GetPos();
ColorU8 dif=vertices[i].GetDif();
pos_buffer.put(pos.GetX());
pos_buffer.put(pos.GetY());
pos_buffer.put(pos.GetZ());
color_buffer.put(dif.GetR());
color_buffer.put(dif.GetG());
color_buffer.put(dif.GetB());
color_buffer.put(dif.GetA());
}
((Buffer)pos_buffer).flip();
((Buffer)color_buffer).flip();
GLShaderFunctions.UseProgram("color");
GLWrapper.glGenBuffers(1, pos_vbo);
GLWrapper.glGenBuffers(1, color_vbo);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*pos_buffer.capacity(),pos_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*color_buffer.capacity(),color_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glGenVertexArrays(1, vao);
GLWrapper.glBindVertexArray(vao.get(0));
//Position attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(0);
GLWrapper.glVertexAttribPointer(0, 3, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*3, 0);
//Color attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(1);
GLWrapper.glVertexAttribPointer(1, 4, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*4, 0);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, 0);
GLWrapper.glBindVertexArray(0);
//Draw
GLWrapper.glBindVertexArray(vao.get(0));
GLWrapper.glEnable(GL4.GL_BLEND);
GLWrapper.glDrawArrays(GL4.GL_LINE_LOOP, 0, 3);
GLWrapper.glDisable(GL4.GL_BLEND);
GLWrapper.glBindVertexArray(0);
//Delete buffers
GLWrapper.glDeleteBuffers(1, pos_vbo);
GLWrapper.glDeleteBuffers(1, color_vbo);
GLWrapper.glDeleteVertexArrays(1, vao);
}
public static void DrawQuadrangle3D(
Vector quadrangle_pos_1,Vector quadrangle_pos_2,
Vector quadrangle_pos_3,Vector quadrangle_pos_4,ColorU8 color) {
Quadrangle quadrangle=new Quadrangle();
Vertex3D[] vertices=new Vertex3D[4];
for(int i=0;i<4;i++) {
vertices[i]=new Vertex3D();
}
vertices[0].SetPos(quadrangle_pos_1);
vertices[1].SetPos(quadrangle_pos_2);
vertices[2].SetPos(quadrangle_pos_3);
vertices[3].SetPos(quadrangle_pos_4);
for(int i=0;i<4;i++) {
vertices[i].SetDif(color);
}
for(int i=0;i<4;i++) {
quadrangle.SetVertex(i, vertices[i]);
}
DrawQuadrangle3D(quadrangle);
}
public static void DrawQuadrangle3D(Quadrangle quadrangle) {
IntBuffer pos_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer color_vbo=Buffers.newDirectIntBuffer(1);
IntBuffer vao=Buffers.newDirectIntBuffer(1);
Vertex3D[] vertices=quadrangle.GetVertices();
FloatBuffer pos_buffer=Buffers.newDirectFloatBuffer(12);
FloatBuffer color_buffer=Buffers.newDirectFloatBuffer(16);
for(int i=0;i<4;i++) {
Vector pos=vertices[i].GetPos();
ColorU8 dif=vertices[i].GetDif();
pos_buffer.put(pos.GetX());
pos_buffer.put(pos.GetY());
pos_buffer.put(pos.GetZ());
color_buffer.put(dif.GetR());
color_buffer.put(dif.GetG());
color_buffer.put(dif.GetB());
color_buffer.put(dif.GetA());
}
((Buffer)pos_buffer).flip();
((Buffer)color_buffer).flip();
GLShaderFunctions.UseProgram("color");
GLWrapper.glGenBuffers(1, pos_vbo);
GLWrapper.glGenBuffers(1, color_vbo);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*pos_buffer.capacity(),pos_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glBufferData(GL4.GL_ARRAY_BUFFER,
Buffers.SIZEOF_FLOAT*color_buffer.capacity(),color_buffer,GL4.GL_STATIC_DRAW);
GLWrapper.glGenVertexArrays(1, vao);
GLWrapper.glBindVertexArray(vao.get(0));
//Position attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, pos_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(0);
GLWrapper.glVertexAttribPointer(0, 3, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*3, 0);
//Color attribute
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, color_vbo.get(0));
GLWrapper.glEnableVertexAttribArray(1);
GLWrapper.glVertexAttribPointer(1, 4, GL4.GL_FLOAT, false, Buffers.SIZEOF_FLOAT*4, 0);
GLWrapper.glBindBuffer(GL4.GL_ARRAY_BUFFER, 0);
GLWrapper.glBindVertexArray(0);
//Draw
GLWrapper.glBindVertexArray(vao.get(0));
GLWrapper.glEnable(GL4.GL_BLEND);
GLWrapper.glDrawArrays(GL4.GL_LINE_LOOP, 0, 4);
GLWrapper.glDisable(GL4.GL_BLEND);
GLWrapper.glBindVertexArray(0);
//Delete buffers
GLWrapper.glDeleteBuffers(1, pos_vbo);
GLWrapper.glDeleteBuffers(1, color_vbo);
GLWrapper.glDeleteVertexArrays(1, vao);
}
public static void DrawSphere3D(Vector center,float radius,int slice_num,int stack_num,ColorU8 color) {
List vertices=new ArrayList<>();
List indices=new ArrayList<>();
int vertex_num=slice_num*(stack_num-1)+2;
//North pole
vertices.add(VectorFunctions.VGet(0.0f, 1.0f, 0.0f));
//Middle
for(int i=1;i vertices=new ArrayList<>();
List indices=new ArrayList<>();
int vertex_num=slice_num*(stack_num-1)+2;
//North pole
vertices.add(VectorFunctions.VGet(0.0f, radius+half_d, 0.0f));
//Middle
for(int i=1;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy