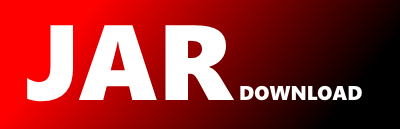
eu.drus.jpa.unit.neo4j.dataset.DatabaseReader Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa-unit-neo4j Show documentation
Show all versions of jpa-unit-neo4j Show documentation
JUnit extension for simple testing of JPA entities and components
The newest version!
package eu.drus.jpa.unit.neo4j.dataset;
import java.sql.Connection;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.ArrayList;
import java.util.Arrays;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import org.jgrapht.Graph;
import org.jgrapht.graph.ClassBasedEdgeFactory;
import org.jgrapht.graph.DefaultDirectedGraph;
public class DatabaseReader {
private GraphElementFactory factory;
public DatabaseReader(final GraphElementFactory factory) {
this.factory = factory;
}
public Graph readGraph(final Connection connection) throws SQLException {
final List nodes = new ArrayList<>();
final List edges = new ArrayList<>();
readGraphElements(connection, edges, nodes);
final DefaultDirectedGraph graph = new DefaultDirectedGraph<>(new ClassBasedEdgeFactory<>(Edge.class));
nodes.forEach(graph::addVertex);
edges.forEach(e -> graph.addEdge(e.getSourceNode(), e.getTargetNode(), e));
return graph;
}
@SuppressWarnings("unchecked")
private void readGraphElements(final Connection connection, final List edgeList, final List nodeList) throws SQLException {
final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy