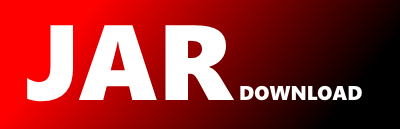
eu.drus.jpa.unit.neo4j.dataset.GraphElement Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa-unit-neo4j Show documentation
Show all versions of jpa-unit-neo4j Show documentation
JUnit extension for simple testing of JPA entities and components
The newest version!
package eu.drus.jpa.unit.neo4j.dataset;
import java.util.Collections;
import java.util.List;
import java.util.stream.Collectors;
public abstract class GraphElement {
private String id;
private List attributes;
private List labels;
protected GraphElement(final String id, final List labels, final List attributes) {
this.id = id;
this.labels = labels;
this.attributes = attributes;
}
public static String toType(final List labels) {
final List tmp = labels.stream().sorted((a, b) -> a.compareTo(b)).collect(Collectors.toList());
return String.join(":", tmp);
}
public List getLabels() {
return Collections.unmodifiableList(labels);
}
public String getType() {
return toType(labels);
}
public String getId() {
return id;
}
public List getAttributes() {
return Collections.unmodifiableList(attributes);
}
public boolean isSame(final GraphElement other, final List attributesToExclude) {
if (!labels.containsAll(other.labels)) {
return false;
}
final List ownAttributes = attributes.stream().filter(a -> !attributesToExclude.contains(a.getName()))
.collect(Collectors.toList());
final List otherAttributes = other.attributes.stream().filter(a -> !attributesToExclude.contains(a.getName()))
.collect(Collectors.toList());
return ownAttributes.containsAll(otherAttributes);
}
}