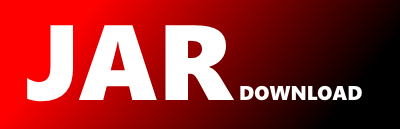
eu.drus.jpa.unit.sql.dbunit.SqlScript Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jpa-unit-rdbms Show documentation
Show all versions of jpa-unit-rdbms Show documentation
Makes JPA Unit support SQL databases
package eu.drus.jpa.unit.sql.dbunit;
import java.util.Iterator;
import java.util.NoSuchElementException;
import java.util.Spliterator;
import java.util.StringTokenizer;
public class SqlScript implements Iterable {
private static final String COMMENT_PATTERN = "(?:--.*)|(?:/\\*(?:[^*]|(?:\\*+[^*/]))*\\*+/)|(?://.*)|(?:#.*)";
private static class SqlScriptIterator implements Iterator {
private final StringTokenizer st;
private String cached;
private boolean hasNextCached;
private boolean hasNext;
private SqlScriptIterator(final String script) {
st = new StringTokenizer(script.replaceAll(COMMENT_PATTERN, ""), ";");
}
@Override
public boolean hasNext() {
return hasNextCached ? hasNext : findNextMatch();
}
@Override
public String next() {
if (hasNext()) {
hasNextCached = false;
return cached;
} else {
throw new NoSuchElementException();
}
}
private boolean findNextMatch() {
boolean match = false;
while (!match && st.hasMoreElements()) {
cached = st.nextToken().trim();
match = !cached.isEmpty();
}
hasNextCached = true;
hasNext = match;
return match;
}
}
private String script;
public SqlScript(final String script) {
this.script = script;
}
@Override
public Iterator iterator() {
return new SqlScriptIterator(script);
}
@Override
public Spliterator spliterator() {
throw new UnsupportedOperationException();
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy