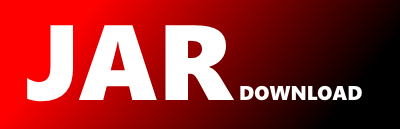
com.github.dakusui.actionunit.actions.Piped Maven / Gradle / Ivy
package com.github.dakusui.actionunit.actions;
import com.github.dakusui.actionunit.Context;
import com.github.dakusui.actionunit.connectors.Connectors;
import com.github.dakusui.actionunit.connectors.Pipe;
import com.github.dakusui.actionunit.connectors.Sink;
import com.github.dakusui.actionunit.connectors.Source;
import com.google.common.base.Function;
import static com.github.dakusui.actionunit.Utils.describe;
import static com.github.dakusui.actionunit.Autocloseables.transform;
import static com.google.common.base.Preconditions.checkNotNull;
import static java.lang.String.format;
import static java.util.Arrays.asList;
import static org.apache.commons.lang3.StringUtils.join;
/**
*
* +--------+ source +---------+
* | With |<>----+------->|Source|
* +--------+ | +---------+
* | |
* | |sinks +---------+
* | +------->|Sink[]|
* A +---------+
* |
* +----------+ pipe +---------+
* |Piped|<>--+------->|Pipe|
* +----------+ | +---------+
* |
* |destinationSinks
* | +---------+
* +------->|Sink[]|
* +---------+
*
*
* @param Input type
* @param Output type
*/
public interface Piped extends With {
Pipe getPipe();
Sink[] getDestinationSinks();
class Impl extends With.Base implements Piped {
protected final Source source;
protected final Pipe pipe;
protected final Sink[] destinationSinks;
private final String sourceName;
private final String pipeName;
private final String destinationSinksName;
@SafeVarargs
public Impl(
final Source source,
final Pipe pipe,
final Sink... destinationSinks) {
this(source, "From", pipe, "Through", destinationSinks, "To");
}
protected Impl(
final Source source, String sourceName,
final Pipe pipe, String pipeName,
final Sink[] destinationSinks, String destinationSinksName) {
this(source, sourceName, pipe, pipeName, Connectors.mutable(), destinationSinks, destinationSinksName);
}
private Impl(
final Source source, String sourceName,
final Pipe pipe, String pipeName,
final Source.Mutable output,
final Sink[] destinationSinks, String destinationSinksName) {
//noinspection unchecked
super(
source,
Named.Factory.create(pipeName,
Sequential.Factory.INSTANCE.create(
asList(
new Tag(0),
new With.Base<>(
output,
Named.Factory.create(
destinationSinksName,
Tag.createFromRange(0, destinationSinks.length)
),
/*(Sink[])*/
destinationSinks)))),
new Sink/**/[] {
new Sink() {
@Override
public void apply(I input, Context context) {
output.set(pipe.apply(input, context.getParent()));
}
public String toString() {
return describe(pipe);
}
}
});
this.source = checkNotNull(source);
this.sourceName = sourceName;
this.pipe = checkNotNull(pipe);
this.pipeName = pipeName;
this.destinationSinks = checkNotNull(destinationSinks);
this.destinationSinksName = destinationSinksName;
}
@Override
public String toString() {
//noinspection unchecked
return format("%s%n%s:%s%n%s:%s%n%s:[%s]",
formatClassName(),
this.sourceName,
describe(this.getSource()),
this.pipeName,
join(transform(
asList(this.getSinks()),
new Function, Object>() {
@Override
public Object apply(Sink sink) {
return describe(sink);
}
}),
","),
this.destinationSinksName,
join(transform(
asList((Sink[]) getDestinationSinks()),
new Function, Object>() {
@Override
public Object apply(Sink input) {
return describe(input);
}
}),
",")
);
}
@Override
public Pipe getPipe() {
return this.pipe;
}
public Sink[] getDestinationSinks() {
return this.destinationSinks;
}
}
enum Factory {
;
@SafeVarargs
public static Piped create(
final Source source,
final Pipe pipe,
final Sink... sinks) {
checkNotNull(source);
checkNotNull(pipe);
//noinspection unchecked
return new Impl<>(source, pipe, sinks);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy