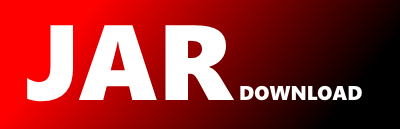
com.github.dakusui.actionunit.actions.ForEach Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of actionunit Show documentation
Show all versions of actionunit Show documentation
A library to build 'action' structure for testing
package com.github.dakusui.actionunit.actions;
import com.github.dakusui.actionunit.core.Action;
import com.github.dakusui.actionunit.core.ValueHandlerActionFactory;
import java.util.Objects;
import java.util.function.Supplier;
import static com.github.dakusui.actionunit.helpers.InternalUtils.describe;
import static com.github.dakusui.actionunit.helpers.InternalUtils.summary;
public interface ForEach extends Action {
Iterable data();
Action createHandler(Supplier data);
Mode getMode();
static ForEach.Builder builder(int id, Iterable extends E> elements) {
return new ForEach.Builder<>(id, elements);
}
class Builder {
private final Iterable extends E> elements;
private final int id;
private Mode mode = Mode.SEQUENTIALLY;
Builder(int id, Iterable extends E> elements) {
this.id = id;
this.elements = Objects.requireNonNull(elements);
}
public Builder sequentially() {
this.mode = Mode.SEQUENTIALLY;
return this;
}
public Builder concurrently() {
this.mode = Mode.CONCURRENTLY;
return this;
}
public ForEach perform(ValueHandlerActionFactory operation) {
Objects.requireNonNull(operation);
//noinspection unchecked
return new ForEach.Impl<>(
id,
operation,
(Iterable) this.elements,
this.mode
);
}
public ForEach perform(Action action) {
Objects.requireNonNull(action);
return perform((factory, data) -> action);
}
}
enum Mode {
SEQUENTIALLY,
CONCURRENTLY
}
class Impl extends ActionBase implements ForEach {
private final ValueHandlerActionFactory handlerFactory;
private final Iterable data;
private final Mode mode;
public Impl(int id, ValueHandlerActionFactory handlerFactory, Iterable data, Mode mode) {
super(id);
this.handlerFactory = Objects.requireNonNull(handlerFactory);
this.data = Objects.requireNonNull(data);
this.mode = Objects.requireNonNull(mode);
}
@Override
public Iterable data() {
return this.data;
}
@Override
public Action createHandler(Supplier data) {
return this.handlerFactory.apply(data);
}
@Override
public Mode getMode() {
return this.mode;
}
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
@Override
public String toString() {
return String.format("%s (%s) %s", super.toString(), mode, summary(describe(this.data)));
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy