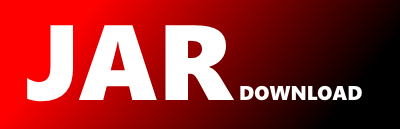
com.github.dakusui.actionunit.actions.While Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of actionunit Show documentation
Show all versions of actionunit Show documentation
A library to build 'action' structure for testing
package com.github.dakusui.actionunit.actions;
import com.github.dakusui.actionunit.core.Action;
import com.github.dakusui.actionunit.core.ActionFactory;
import com.github.dakusui.actionunit.core.Context;
import java.util.Objects;
import java.util.function.Predicate;
import java.util.function.Supplier;
public interface While extends Action, Context {
Supplier value();
Predicate check();
Action createAction();
class Builder {
private final Predicate check;
private final Supplier value;
private final int id;
public Builder(int id, Supplier value, Predicate check) {
this.id = id;
this.value = Objects.requireNonNull(value);
this.check = Objects.requireNonNull(check);
}
public While perform(ActionFactory actionFactory) {
return new Impl<>(id, value, check, Objects.requireNonNull(actionFactory));
}
public While perform(Action action) {
return perform(self -> action);
}
}
class Impl extends ActionBase implements While {
private final Supplier value;
private final Predicate check;
private final ActionFactory actionFactory;
Impl(int id, Supplier value, Predicate check, ActionFactory actionFactory) {
super(id);
this.value = value;
this.check = check;
this.actionFactory = actionFactory;
}
@Override
public Supplier value() {
return value;
}
@Override
public Predicate check() {
return check;
}
@Override
public Action createAction() {
return actionFactory.get();
}
@Override
public void accept(Visitor visitor) {
visitor.visit(this);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy