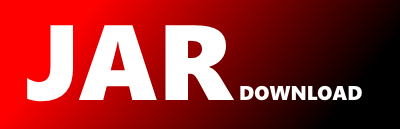
com.github.dakusui.cmd.core.Tee Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of cmd Show documentation
Show all versions of cmd Show documentation
Command line streamer library for Java 8
package com.github.dakusui.cmd.core;
import java.util.*;
import java.util.concurrent.ArrayBlockingQueue;
import java.util.concurrent.ForkJoinPool;
import java.util.concurrent.TimeUnit;
import java.util.concurrent.atomic.AtomicInteger;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
import java.util.stream.Stream;
import java.util.stream.StreamSupport;
import static java.util.stream.Collectors.toList;
public class Tee extends Thread {
private static final Object SENTINEL = new Object() {
@Override
public String toString() {
return "SENTINEL";
}
};
private final Stream in;
private final List> queues;
private final List> streams;
Tee(Stream in, int numDownStreams, int queueSize) {
this.in = Objects.requireNonNull(in);
this.queues = new LinkedList<>();
for (int i = 0; i < numDownStreams; i++) {
this.queues.add(new ArrayBlockingQueue<>(queueSize));
}
this.streams = createDownStreams();
}
@SuppressWarnings("WeakerAccess")
public List> streams() {
return Collections.unmodifiableList(this.streams);
}
@Override
public void run() {
List> pendings = new LinkedList<>();
Stream.concat(in, Stream.of(SENTINEL))
.forEach((Object t) -> {
pendings.addAll(this.queues);
synchronized (this.queues) {
while (!pendings.isEmpty()) {
this.queues.stream()
.filter(pendings::contains)
.filter(queue -> queue.offer(t))
.forEach(pendings::remove);
this.queues.notifyAll();
try {
this.queues.wait();
} catch (InterruptedException ignored) {
}
}
}
});
}
public static Connector tee(Stream in) {
return new Connector<>(in);
}
public static Connector tee(Stream in, int queueSize) {
return tee(in).setQueueSize(queueSize);
}
private List> createDownStreams() {
return queues.stream()
.map((Queue
© 2015 - 2025 Weber Informatics LLC | Privacy Policy